
April 18, 2024 08:36 by
Peter
As a potent framework for creating dynamic and interactive single-page applications (SPAs), Angular has made a name for itself. An important problem for developers working with Angular apps is effectively and efficiently moving data across components. Fortunately, Angular offers a number of techniques, each designed to fit a particular use case or scenario, to help with this data transmission process. We'll examine the many Angular methods of data transfer across components in this article.
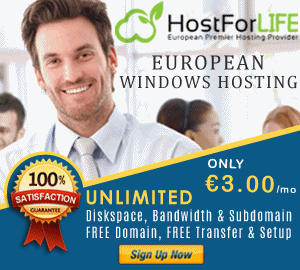
1. Bindings for Input and Output
Angular components can employ input and output bindings to exchange data with one another. While output bindings allow child components to broadcast events back to their parent components, input bindings let a parent component to pass data to its child components.
// Parent Component
import { Component } from '@angular/core';
@Component({
selector: 'app-parent',
template: `
<app-child [inputData]="parentData" (outputEvent)="handleOutput($event)"></app-child>
`
})
export class ParentComponent {
parentData = 'Data from parent';
handleOutput(data: any) {
// Handle emitted data from child component
}
}
// Child Component
import { Component, Input, Output, EventEmitter } from '@angular/core';
@Component({
selector: 'app-child',
template: `
<p>{{ inputData }}</p>
<button (click)="emitData()">Emit Data</button>
`
})
export class ChildComponent {
@Input() inputData: any;
@Output() outputEvent = new EventEmitter<any>();
emitData() {
this.outputEvent.emit('Data from child');
}
}
2. Services
Angular services act as singletons that can be injected into any component throughout the application. They are ideal for sharing data and functionality across multiple components.
// Shared Service
import { Injectable } from '@angular/core';
import { BehaviorSubject } from 'rxjs';
@Injectable({
providedIn: 'root'
})
export class DataService {
private dataSubject = new BehaviorSubject<any>(null);
data$ = this.dataSubject.asObservable();
setData(data: any) {
this.dataSubject.next(data);
}
}
// Components
import { Component } from '@angular/core';
import { DataService } from './data.service';
@Component({
selector: 'app-sender',
template: `
<button (click)="sendData()">Send Data</button>
`
})
export class SenderComponent {
constructor(private dataService: DataService) {}
sendData() {
this.dataService.setData('Data from sender');
}
}
@Component({
selector: 'app-receiver',
template: `
<p>{{ receivedData }}</p>
`
})
export class ReceiverComponent {
receivedData: any;
constructor(private dataService: DataService) {
this.dataService.data$.subscribe(data => {
this.receivedData = data;
});
}
}
3. Route Parameters and Query Parameters
// Routing Module
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
import { Component1Component } from './component1.component';
import { Component2Component } from './component2.component';
const routes: Routes = [
{ path: 'component1/:id', component: Component1Component },
{ path: 'component2', component: Component2Component }
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
// Components
import { Component, OnInit } from '@angular/core';
import { ActivatedRoute } from '@angular/router';
@Component({
selector: 'app-component1',
template: `
<p>{{ id }}</p>
`
})
export class Component1Component implements OnInit {
id: string;
constructor(private route: ActivatedRoute) {}
ngOnInit() {
this.route.params.subscribe(params => {
this.id = params['id'];
});
}
}
@Component({
selector: 'app-component2',
template: `
<a [routerLink]="['/component1', '123']">Go to Component 1 with ID</a>
`
})
export class Component2Component { }
Data communication between components is essential to creating scalable and reliable solutions in Angular apps. Through the utilization of methods like services, routing systems, and input and output bindings, developers may proficiently oversee data flow and communication in their applications. Gaining knowledge of these Angular data transfer methods enables developers to create Angular apps that are more modular, efficient, and manageable.