
November 13, 2014 10:34 by
Peter
Sometimes we would like to do tricks with the Crystal Reports 2013 engine, like taking advantage of the exporting functions to make Word, Excel, or PDF files. What if we do not scan the data from a database? this article shows the details on making a Crystal Reports sub report, and the way to fill it from code, without a database.
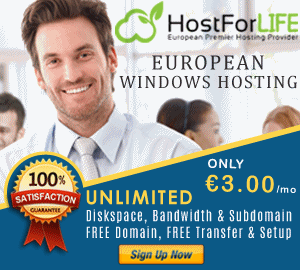
Using the code
- First thing you should do is Create the Project: File -> New -> New Project
- Then, creat a new Crystal Reports Visual Basic Windows Application.
- Next, Create the DataSet schema. Right click the Crystal Reports project -> Add -> New Folder. Name it that folder: “DataSets”.
- Right click the “DataSets” folder , click Add then select New Item.
- Now, create the new CRDataSet DataSet. Right click the CRDataSet Designer Page, click Add, then select New element. I give it a name: MainTable.
- Add new Field1, Field2, Field3 rows.
- Repeat steps three and four, then Add a new DetailTable element.
You will have the following DataSet tables schema as shown as picture below:
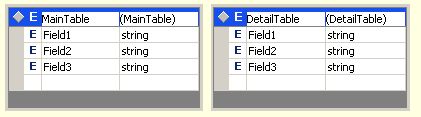
Now, we are going to Create report on Crystal Report:
1. First, Right click the Crystal Reports project -> Click “Add” -> SelectNew Folder.
2. Name it that folder “Reports”.
3.Right click on the Reports folder -> Click Add -> then select New Item.
4. Open a new MainReport Crystal Reports report.
5. Now, Click the Register Later button to close the register window, if it appears.
6. Select the Subreport and then click OK button. In the Contain Subeport Expert window Data tab, expand the Project Data -> Select ADO.NET DataSet -> CrystalReport.CRDataSet.
7. Choose MainTable. Now, Click the Insert Table button.
8. Click Next.
9. Next Step: Click the Add All button -> Next -> Next -> Next.
10. Next to the Create a Subreport choice of the Subreport window tab, then fill DetailReport in the Report Name edit box.
11. Click the Report Expert button. Insert the DetailTable. Then Click Next to open the Subreport Generator. Add All fields. Click Next. Click Next. Click Next. Click Next.
12. Type "No DB Subreport Crystal Report Sample" as the Subreport Title.
13. Click Finish.
14. After that Click Next. Type "No DB Crystal Report Sample." as the Main Report title. And now, click Finish.
And now we will add the a CrystalReportViewer1 to the form.
- Open the Form1 form. Click View -> Toolbox in the menu.
- Drag a CrystalReportViewer into the form.
- Right click the CrystalReportViewer1 CrystalReportViewer in the form -> Properties.
- Set the Dock property to Fill.
- Next step, Add the code to fill & show the Crystal Reports report. Then, Right click the Form -> View Code. Add the following Form1_Activated method:
Private Sub Form1_Activated(ByVal sender As Object, _
ByVal e As System.EventArgs) _
Handles MyBase.Activated
Dim dsObj As CRDataSet = New CRDataSet
FillDataSet(dsObj)
Dim cr As MainReport = New MainReport
' Set the report DataSet
cr.SetDataSource(dsObj)
CrystalReportViewer1.ReportSource = cr
End Sub
Now, I’m gonna Add the following FillDataSet code:
Public Sub FillDataSet(ByRef dataSet As CRDataSet)
' Turn off constraint checking before the dataset is filled.
' This allows the adapters to fill the dataset without concern
' for dependencies between the tables.
dataSet.EnforceConstraints = False
Try
' Attempt to fill the dataset MainTable
dataSet.MainTable.AddMainTableRow("Hello!", _
"This is", "my sample data")
' Attempt to fill the dataset DetailTable
dataSet.DetailTable.AddDetailTableRow("This is", _
"the detail", "information")
dataSet.DetailTable.AddDetailTableRow("added", _
"without the", "need of")
dataSet.DetailTable.AddDetailTableRow("actually", _
"access a", "database.")
Catch ex As Exception
' Add your error handling code here.
Throw ex
Finally
' Turn constraint checking back on.
dataSet.EnforceConstraints = True
End Try
End Sub
Build and Run this Application. Hope this tutorial works for you!