In contemporary web development, logging plays a crucial role in program monitoring and troubleshooting. Node.js offers a variety of logging tools for monitoring and analyzing application operations because of its event-driven architecture. This article will walk through how to build a logging system for a Node.js application using Winston, a versatile logging package, and Express, a popular web framework.
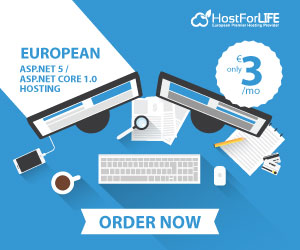
What is Logging?
The process of keeping track of activities and occurrences inside a software system is called logging. These log entries help with debugging, monitoring, auditing, and security. They include timestamps, severity levels, messages, and contextual data. Logging helps with performance analysis, intrusion detection, compliance adherence, and troubleshooting by capturing important information about system behavior. With their ability to provide insights into application flow, performance bottlenecks, user actions, and security concerns, logs are an indispensable tool for developers, administrators, and security professionals. Logging is essentially a basic procedure used in software development and operations that makes software systems easier to manage, secure, and monitor.
Why we use Logging?
Logging serves several essential purposes in software development:
- Debugging and Troubleshooting: Logs are an important source of information for troubleshooting and identifying difficulties with a program. To comprehend the execution flow, spot faults, and find the source of problems, developers can go through log messages.
- Monitoring and Performance Optimization: Developers may keep an eye on an application's performance in real time with the help of logs. Developers can pinpoint performance bottlenecks, maximize resource use, and raise the application's general effectiveness by examining log data.
- Auditing and Compliance: Keeping track of user actions and system events is facilitated by logging. For compliance with legal standards like GDPR, HIPAA, or PCI DSS, this audit trail is crucial. Logs are useful for monitoring user behavior, spotting illegal access, and guaranteeing the accuracy of data.
- Security and Intrusion Detection: When it comes to identifying security risks and unauthorized access attempts, logs are essential. Events including unsuccessful login attempts, questionable activity, and possible security breaches are recorded in security logs. Administrators are able to quickly identify and address security incidents by examining security logs.
Steps for Creating a Project
Step 1. Setting Up the Project
Use my previous article for setting up Node.js, "How to upload file in Node.js" In this article, we mentioned important commands for uploading files in Node.js.
Step 2. Integrating Winston for Logging
Winston is a versatile Node.js logging package that supports many transports, such as file, database, and terminal. Winston will be set up to log messages to both the console and a log file at various levels (info, error, etc.).
// main.js
const winston = require('winston');
// Create a logger instance
const logger = winston.createLogger({
level: 'info', // Logging level
format: winston.format.json(), // Log format
transports: [
// Log to the console
new winston.transports.Console(),
// Log to a file
new winston.transports.File({ filename: 'logging_file.log' })
]
});
Step 3. Implementing Logging Middleware in Express
We are able to intercept and handle incoming requests thanks to express middleware. A unique middleware function will be developed to log incoming HTTP requests. Request methods, URLs, and other pertinent data will be recorded by this middleware and logged using Winston.
// Middleware to log requests
app.use((req, res, next) => {
logger.log('info', `${req.method} ${req.url}`);
next();
});
Step 4. Creating Routes and Handling Errors
After installing logging middleware, we will create our Express application's routes. To show how to handle requests, we'll construct a simple route for the main page ("/"). Furthermore, error handling middleware will be implemented to log and manage any faults that arise during request processing.
// Route handling
app.get('/', (req, res) => {
res.send('Hello, world!');
});
// Error handling middleware
app.use((err, req, res, next) => {
logger.log('error', err.stack);
res.status(500).send('Something broke!');
});
Step 5. Serving Log File via Web Interface
We'll develop an additional route ("/logs") that reads the contents of the log file and sends it to the client in order to give a user-friendly way for log files to be viewed. In order to ensure security and avoid dangerous infinite loops, this route will ignore logging requests so they are not kept in the log file.
// Route to serve log file
app.get('/logs', (req, res) => {
fs.readFile('combined.log', 'utf8', (err, data) => {
if (err) {
logger.log('error', err);
return res.status(500).send('Error reading log file');
}
res.type('text/plain').send(data);
});
});
Step 6. Running the Application
Finally, let us launch our Express application and verify its operation. To start the HTTP request logging, start the server and visit the home page. You may also view the contents of the log file using the web interface by going to the "/logs" route.
node main.js
Output
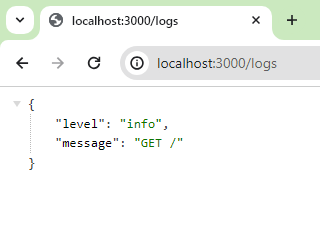