
April 3, 2024 09:51 by
Peter
One of the main components of the Angular framework is Angular directives, which are used to attach to the DOM, change elements, and enhance the functionality and structure of HTML.
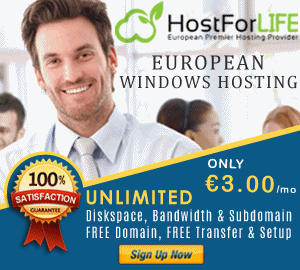
Predefined directives with an angle
ngStyle, ngClass, ngIf, ngFor, and ngswitch
Why are the custom directives being created?
When developing real-time applications, it's common to need to preserve consistency and reusability while utilizing the same feature repeatedly.
As an illustration
Only allow numbers in the registration form; any text entered by the user will be blocked by the input field.
Make the special directive.

ng g directive Directive/AollwNumber
AllowNumber.ts
import { Directive, ElementRef, HostListener } from '@angular/core';
@Directive({
selector: '[appAllowNumber]'
})
export class AllowNumberDirective {
// Allow numbers.
private regex: RegExp = new RegExp(/^\d+$/);
// Allow key codes for special events. Reflect :
// Backspace, tab, end, home
private specialKeys: Array<string> = ['Backspace', 'Tab', 'End', 'Home', 'ArrowLeft', 'ArrowRight', 'Delete'];
constructor(private el: ElementRef) {
}
@HostListener('keydown', ['$event'])
onKeyDown(event: KeyboardEvent) {
debugger
const selecetdText = window.getSelection().toString();
// Allow Backspace, tab, end, and home keys
if (this.specialKeys.indexOf(event.key) !== -1) {
return;
}
let current: string = this.el.nativeElement.value;
if(selecetdText != "" && current.indexOf(selecetdText) !== -1){
current = current.slice(0,current.indexOf(selecetdText));
}
// We need this because the current value on the DOM element
// is not yet updated with the value from this event
let next: string = current.concat(event.key);
if (next && !String(next).match(this.regex)) {
event.preventDefault();
} else if (event.currentTarget['max'] != "undefined" && event.currentTarget['max'] != "" && event.currentTarget['max'] != null && !(Number(event.currentTarget['max']) >= Number(next))) {
event.preventDefault();
}
if(event.currentTarget['min'] != "undefined" && event.currentTarget['min'] != "" && event.currentTarget['min'] != null && !(Number(event.currentTarget['min']) <= Number(next)) ){
event.preventDefault();
}
}
/**
* This is for restrict paste value in input field
* @param e
*/
@HostListener('paste', ['$event']) blockPaste(e: KeyboardEvent) {
e.preventDefault();
}
}
Register the directive App module
After the creation of the directive, this is required to be registered in the root directive module or app module.
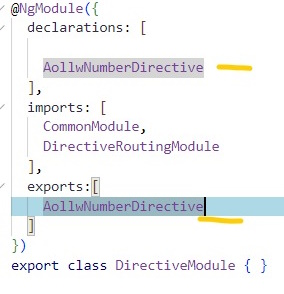
Decore directive in the Input Field
