The most difficult part in writing AJAX code is to handle errors and exceptions. Session timeout is one of the most trivial error that one needs to consider while creating an AJAX enabled application. In this post, I will show you the way of handling session timeout errors server HTTP errors in AJAX.
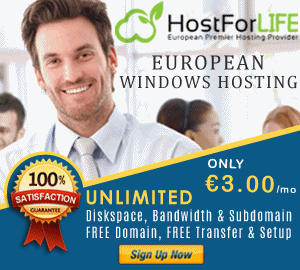
Normally, we use AJAX to deliever HTML content that we put in a DIV or SPAN tag and display it on screen. But suppose the request that we sent using AJAX was generated an error due to session timeout then the session timeout screen will be shown in DIV tag. Any error generated by server let say http 404 error or http 505 can lead to display such error in our display DIV.
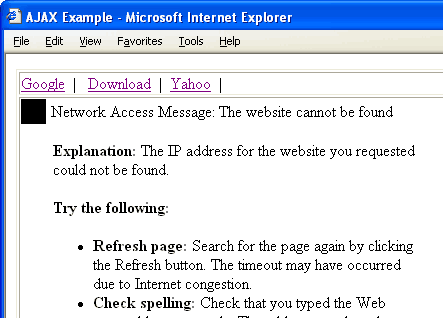
How to Handle Session Timeout Errors
One solution that you can use to handle the session timeout errors is using JSON or XML format as output for your AJAX requests. You have to check for session in your script, say for example if you are using JSP then probably you can do something like this:
if(null == session || session.getAttribute("SOME_ATTR_THAT_I_PLACED_IN_SESSION")) {
isSessionNull = true;
}
...
{
<%
if(isSessionNull) {
out.println("status: error");
}
else {
out.println("status: success");
}
%>
}
In short, create a status field in your JSON script or a status tag in your XML which will describe the status of the request. I am looking for some more ways of checking session timeouts in AJAX. Kindly let me know if you have a good solution.
Handle Server HTTP errors in AJAX
HTTP errors like 404, 503 are sometimes tedious to handle using AJAX. There is a simple trick to solve this problem. First let's see simple AJAX handler function that you use to get response XML/text through AJAX.
xhr.open("GET", "http://someurl", true);
xhr.onreadystatechange = handleHttpResponse;
xhr.send(null);
...
...
function handleHttpResponse() {
if (xhr.readyState == 4) {
//handle response your way
spanBodyContent.innerHTML = xhr.responseText;
}
}
Normal AJAX handlers has a if to check readyState and if it is 4 (response arrived state) then you will get the response from xhr.responseText or xhr.responseXML.
AJAX’s XMLHttpRequest object has attributes to check the status of your http request. You can use two attributes: status and statusText for knowing this.
xhr.status
..
xhr.statusText
Thus by checking status attribute from your AJAX xhr object can solve your problem.
xhr.open("GET", "http://someurl", true);
xhr.onreadystatechange = handleHttpResponse;
xhr.send(null);
...
...
function handleHttpResponse() {
if (xhr.readyState == 4) {
try {
if(xhr.status == 200) {
//handle response your way
spanBodyContent.innerHTML = xhr.responseText;
}
}catch(e) {//error}
}
}
At the end, if status is not 200, you may not want to update your DOM with the ajax result and display some error message on your web page.
HostForLIFE.eu AJAX Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
