
November 3, 2023 08:14 by
Peter
The Repository Pattern is a popular design pattern for separating data access and manipulation logic from the rest of the application. While the Repository Pattern is more frequently linked with backend or server-side development, you can still use a variant of it in Angular for data management. Here's an example of how to use Angular to construct a simplified version of the Repository Pattern:
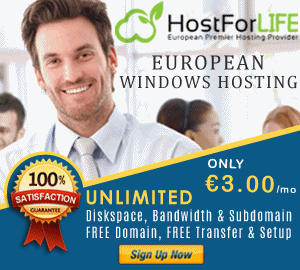
Make a Repository
To handle data operations, create a repository. Create a file called user.repository.ts with the following code:
import { Injectable } from ‘@angular/core’;
import { HttpClient } from ‘@angular/common/http’;
import { Observable } from ‘rxjs’;
import { User } from ‘./user.model’;
@Injectable({
providedIn: ’ root ’ ,
})
export class UserRepository {
private apiUrl = ‘https://api.example.com/users’;
constructor(private http: HttpClient) {}
getAllUsers(): Observable<User[]>{
return this.http.get<User[]>(this.apiUrl);
}
getUserById(id:number): Observable<User>{
return this.http.get<User>(‘${this.apiUrl}/${id}’);
}
createUser(user:User): Observable<User>{
return this.http.post<User>(this.apiUrl,user);
}
updateUser(user:User): Observable<User>{
return this.http.put<User>(>(‘${this.apiUrl}/${user.id}’, user);
}
deleteUser(id:number): Observable<any>{
return this.http.delete(>(‘${this.apiUrl}/${id}’);
}
}
Use the Repository in a Component
Make a component that makes use of the repository for data operations. Create a file called user.component.ts with the following code:
import { Component,OnInit } from ‘@angular/core’;
import { User } from ‘./user.model’;
import { UserRepository } from ‘./user.repository’;
@Component({
selector: ‘app-user’,
template:’
<h2>User Component</h2>
<ul>
<li *ngFor=”let user of users”>{{ user.name}}</li>
</ul>
})
export class UserComponent implements OnInit {
users:User[];
constructor( private userRepository: UserRepository) {}
ngOnInit(): void {
this.loadUsers();
}
loadUsers():void{
this.userRepository.getAllUsers().subscribe((users:User[]) => {
this.users = users;
});
}
}
Activate the Repository
Import the UserRepository into the app.module.ts file. Include it in the providers array of the @NgModule decorator. As an example:
import { NgModule } from ‘@angular/core’;
import { BrowserModule } from ‘@angular/platform-browser’;
import { HttpClientModule } from ‘@angular/common/http’;
import { UserRepository } from ‘./user.repository’;
import { UserComponent } from ‘./user.component’;
@NgModule({
declarations: [UserComponent],
imports : [BrowserModule,HttpClientModule],
providers : [UserRepository],
bootstrap : [UserComponent],
})
export class AppModule {}
Create and launch the program
To build and serve the Angular application, use the following command:
ng provide
Your app will be available at http://localhost:4200.
The UserRepository in this example encapsulates the data operations for managing users. The repository is used by the UserComponent by injecting it into its constructor and using its methods to retrieve user data.
You separate the code for data access and manipulation from the components by using this simplified version of the Repository Pattern in Angular. This encourages code reuse, testability, and maintainability while also providing a clear interface for working with the data layer.