In this blog post, we'll go over how to build an Angular application that allows users to directly capture photographs from their webcams. To accomplish this, we'll use the ngx-webcam library, which includes webcam capabilities and covers the library's installation as well as the basic setup of the Angular application in the project.
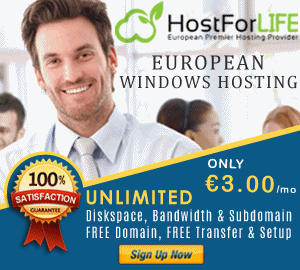
Step 1: Begin by creating a new Angular project.
If you haven't previously, enter the following command in your terminal or command line to install the Angular CLI globally:
npm install -g @angular/cli
Make a new Angular project as follows:
ng new CaptureImage
Go to the project directory:
cd D:\Angular\Project\CaptureImage
Step 2: Set up the ngx-webcam Library.
npm install ngx-webcam
Step 3: Incorporate ngx-webcam into your Angular app.
Import the WebcamModule from ngx-webcam into the src/app/app.module.ts file:
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { ImageWebcamComponent } from './image-webcam/image-webcam.component';
import { WebcamModule } from 'ngx-webcam';
@NgModule({
declarations: [
AppComponent,
ImageWebcamComponent,
],
imports: [
BrowserModule,
AppRoutingModule,
WebcamModule,
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Step 4: Make a Webcam Component
Make a new component to manage webcam functionality. Run the following command in your terminal:
ng generate component image-webcam
Open src/app/image-webcam/image-webcam.component.ts and add the following logic to capture images:
import { Component } from '@angular/core';
import { Observable, Subject } from 'rxjs';
import { WebcamImage, WebcamInitError, WebcamUtil } from 'ngx-webcam';
@Component({
selector: 'app-image-webcam',
templateUrl: './image-webcam.component.html',
styleUrls: ['./image-webcam.component.css']
})
export class ImageWebcamComponent {
private trigger: Subject<any> = new Subject();
webcamImage: any;
private nextWebcam: Subject<any> = new Subject();
sysImage = '';
ngOnInit() {}
public getSnapshot(): void {
this.trigger.next(void 0);
}
public captureImg(webcamImage: WebcamImage): void {
this.webcamImage = webcamImage;
this.sysImage = webcamImage!.imageAsDataUrl;
console.info('got webcam image', this.sysImage);
}
public get invokeObservable(): Observable<any> {
return this.trigger.asObservable();
}
public get nextWebcamObservable(): Observable<any> {
return this.nextWebcam.asObservable();
}
}
Add the following code to src/app/image-webcam/image-webcam.component.html.
<div class="container mt-5">
<h2>Angular Webcam Capture Image from Camera</h2>
<div class="col-md-12">
<webcam
[trigger]="invokeObservable"
(imageCapture)="captureImg($event)"
></webcam>
</div>
<div class="col-md-12">
<button class="btn btn-danger" (click)="getSnapshot()">
Capture Image
</button>
</div>
<div class="col-12">
<div id="results">Your taken image manifests here...</div>
<img [src]="webcamImage?.imageAsDataUrl" height="400px" />
</div>
</div>
Step 5: Incorporate the Webcam Component
Add the following code to src/app/app.component.html:
<app-image-webcam></app-image-webcam>
<router-outlet></router-outlet>
Step 6: Execute your Angular Application
ng ng serve -o
Your Angular application should now have webcam capabilities. This section covers the fundamentals of integrating the ngx-webcam library into an Angular project.
If you run into any problems or have any additional questions, please let me know and I'll be happy to help.
Thank you for reading, and I hope this post has given you a better knowledge of how to use ngx-webcam to capture webcam images in Angular.
"Keep coding, innovating, and pushing the limits of what's possible."
Have fun coding.