In this article, we will look at the fundamentals of Angular components, examining their importance and the important features that make them necessary for modern web development. By the conclusion, you'll have a firm understanding of Angular's components.
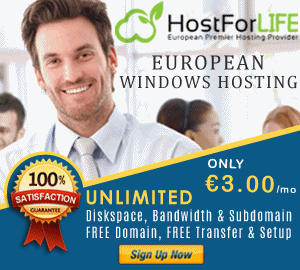
Google's Angular is a popular open-source web application framework for developing dynamic web apps. The concept of components, which serve as the framework's building blocks, is central to Angular. It's commonly used to create dynamic, single-page web apps. The component-based architecture of Angular is one of its primary characteristics.
A component is an essential component of a user interface. It is a reusable and modular structure that wraps a portion of the application's functionality and user interface. Components are in charge of establishing the structure of a UI component and managing the logic associated with that component. Each Angular component is made up of a TypeScript class and a template.
To create a new component, use the following command. Change "my-component" to whatever name you desire for your component.
ng generate component my-component
Components in Angular serve multiple functions, and their utilization is critical to the framework's architecture. Here's why components are so important in Angular development:
- Modularity is promoted via components, which divide down the user interface into smaller, reusable, and manageable sections. Each component contains a specific portion of the user interface and its accompanying logic.
- Components can be reused across multiple portions of the program, making code maintenance and updating easy. Reusable components help to make the development process more efficient and scalable.
- Components improve code readability and maintainability by grouping it into logical and self-contained sections. This organizational structure facilitates developers' understanding, maintenance, and collaboration on the codebase.
- Data Binding: Components enable sophisticated data binding techniques, enabling for smooth data and user interface synchronization. This streamlines the handling of user input and application state updates.
- Components include lifecycle hooks, which allow developers to access various stages of a component's life. This is useful for tasks such as initialization, cleanup, and responding to changes.
- Components adhere to the idea of separation of concerns by splitting the application logic into discrete sections. This division facilitates the management and testing of various components of the application.
An Angular component's main characteristics
1. Typescript Component Class
The component class is built in TypeScript and contains the component's logic.
It often includes attributes and methods that specify the component's behavior.
The component class's properties can be tied to the component's template, allowing for dynamic modifications.
Example
// app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'My Angular App';
// Other properties and methods can be defined here
}
2. Decorator of Components
The '@Component' decorator is used to define the component's metadata. The selector, template, and styles are all part of this. The'selector' is a CSS selector that identifies a template component. It is used to incorporate the component into other templates. The 'templateUrl' indicates the location of the component's HTML template file.
The array'styleUrls' contains URLs to external style sheets that will be applied to the component.
3. HTML template
The template is an HTML file that defines the structure of the view of the component. To improve the dynamic behavior of the UI, Angular uses a specific vocabulary in templates, including data binding, directives, and other capabilities.
Example
<!-- app.component.html -->
<h1>{{ title }}</h1>
<p>This is my Angular application.</p>
4. CSS/SCSS Styles
The styles define the component's look.
Styles can be defined directly in the '@Component' decorator's'styles' property or in external style sheets referenced via the'styleUrls' property.
Example
/* app.component.css */
h1 {
color: blue;
}
5. Integration of Modules
Components must be included in an Angular module. The module specifies which components are associated with it.
The 'declarations' array in the module metadata lists all of the module's components, directives, and pipes.
Example
// app.module.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppComponent } from './app.component';
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule],
bootstrap: [AppComponent]
})
export class AppModule {}
6. Data Binding
Components can communicate with the template using data binding. There are different types of data binding in Angular, including one-way binding ( '{{ expression }} ') property binding ( '[property]="expression" '), and event binding ( '(event)="handler" ').
Example
<!-- app.component.html -->
<p>{{ title }}</p>
<button (click)="changeTitle()">Change Title</button>
' ' '
' ' 'typescript
// app.component.ts
export class AppComponent {
title = 'My Angular App';
changeTitle() {
this.title = 'New Title';
}
}
In conclusion, Angular components are essential for developing modular and maintainable apps. They are made up of a TypeScript class that has been annotated with a decorator that provides metadata such as selector, template, and styles. The structure is defined by the template, the appearance by the styles, and the logic and data are handled by the component class.
If you run into any problems or have any additional questions, please let me know and I'll be happy to help.
Thank you for reading, and I hope this post has helped you gain a better grasp of Angular Components.
"Keep coding, innovating, and pushing the limits of what's possible."
Have fun coding.