This article covers fundamental file operations in node.js, including Reading, Writing, Updating, and Deleting files. Whether it involves accessing configuration files, processing user-uploaded files, or persisting data to disc, file management is an integral part of many applications. Node.js's "fs" (file system) module is indispensable for streamlining file-handling procedures. It provides a vast array of functions and methods for completing various file-related tasks.
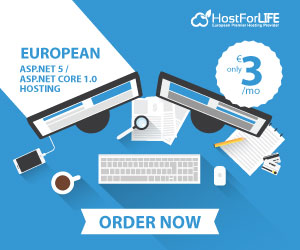
Node.js operation using file management
- Creating File Reading File
- Modifying File
- Delete File
How do you create a Node.js file?
Node.js offers a rapid and efficient method for creating files, which is a necessary task for many applications. In this post, we will examine a variety of synchronous and asynchronous Node.js file generation methods. We will discuss how to use the fs (file system) module, how to handle errors, and best practices for effective file creation. At the conclusion of this article, you will have a comprehensive comprehension of how to create and write files in Node.js, enabling you to handle file-related tasks competently.
Code
const fs = require('fs');
// Specify the file path and content
const filePath = 'path/to/newFile.txt';
const fileContent = 'This is the content of the new file.';
// Asynchronous file creation and writing
fs.writeFile(filePath, fileContent, 'utf8', (err) => {
if (err) {
console.error('Error creating file:', err);
return;
}
console.log('File is created successfully.');
});
// Synchronous file creation and writing
try {
fs.writeFileSync(filePath, fileContent, 'utf8');
console.log('File created successfully.');
} catch (err) {
console.error('Error creating file:', err);
}
Explanation
Using the writeFile method, a file can be created asynchronously by providing the file path, the content, the encoding, and a callback function to manage success or failure. A try-catch block is used to manage errors when using the writeFileSync method to create and write a file synchronously.
How is a file read in Node.js?
Reading a file in Node.js involves obtaining access to and retrieving the file's contents from the file system. It necessitates reading file data using the asynchronous or synchronous readFile or readFileSync methods of the fs module. Asynchronous reading permits non-blocking I/O operations while synchronous reading prevents code execution. Error management is essential for addressing issues such as file not found and permissions errors. Applications that require file-based data processing, such as reading configuration files, parsing user uploads, or extracting data from databases, must have the capability to read files.
Code
const fs = require('fs');
// Specify the file path
const filePath = 'path/to/file.txt';
// Asynchronous file reading
fs.readFile(filePath, 'utf8', (err, data) => {
if (err) {
console.error('Error reading the file:', err);
return;
}
console.log('File contents:', data);
});
// Synchronous file reading
try {
const data = fs.readFileSync(filePath, 'utf8');
console.log('File contents:', data);
} catch (err) {
console.error('Error reading the file:', err);
}
Explanation
The code shows how to read files in Node.js. ReadFile is used for asynchronous reading, and a callback function is used for error handling. In a try-catch block, readFileSync is used for synchronous reading. If no errors happen, both techniques log file contents. While synchronous reading halts code execution until the file is read, asynchronous reading permits parallel tasks.
How to update a file in Node.js?
In the context of Node.js, updating a file is the act of editing or adding data to an already-existing file on the file system. It entails opening the file with the fs (file system) module, making the required adjustments, and then writing the revised content back into the file. A file can be updated by performing operations like replacing certain lines or entries, appending new data at the end, or replacing specific blocks of text. The usual procedure for this is to read the data in the file, update or add to it as necessary, and then write the updated data back into the file. Developers can dynamically update the contents of files, maintain data integrity, and reflect the most recent information in applications that rely on file-based data storage by updating files in Node.js.
Code
const fs = require('fs');
// Specify the file path
const filePath = 'path/to/file.txt';
// Specify the updated content
const updatedContent = 'This is the updated content of the file.';
// Asynchronous file updating
fs.writeFile(filePath, updatedContent, 'utf8', (err) => {
if (err) {
console.error('Error updating the file:', err);
return;
}
console.log('File updated successfully.');
});
// Synchronous file updating
try {
fs.writeFileSync(filePath, updatedContent, 'utf8');
console.log('File updated successfully.');
} catch (err) {
console.error('Error updating the file:', err);
}
Explanation
The file location, the modified content, and optional encoding are passed to the writeFile method, which is used to update the file asynchronously. The identical operation is carried out synchronously using the writeFileSync function. Try-catch blocks are used for error handling, and success or error messages are logged appropriately.
How to delete a file in Node.js?
The fs (file system) module is used in Node.js to delete files by deleting them from the file system. Applications frequently use it to perform resource cleanup, temporary file deletion, and file storage management. The fs.unlink method is offered by Node.js for asynchronous file deletion. This method runs a command using the file path as an argument function when the deletion is finished or if there is a problem. To handle situations when the file cannot be erased because of permissions or if the file doesn't exist, error handling is essential. Node.js has fs.unlinkSync for synchronous file deletion, but due to its blocking nature, it should only be used in extreme cases. Developers may effectively manage files, ensure that unwanted or superfluous files are removed from the file system, and maintain a tidy and organized file storage environment by utilizing Node.js's file deletion capabilities.
Code
const fs = require('fs');
// Specify the file path
const filePath = 'path/to/file.txt';
// Asynchronous file deletion
fs.unlink(filePath, (err) => {
if (err) {
console.error('Error deleting the file:', err);
return;
}
console.log('File deleted successfully.');
});
// Synchronous file deletion
try {
fs.unlinkSync(filePath);
console.log('File deleted successfully.');
} catch (err) {
console.error('Error deleting the file:', err);
}
Explanation
First, require("fs") is used to import the fs module. The file path and a callback function are passed to the unlink method as its first and second arguments, respectively, before the file is asynchronously deleted. Whenever something is finished or if there is an error, the callback function is called. The callback console is used for handling errors. By means of console.log(), success and errors are recorded.
The synchronous variant of unlink is the unlinkSync function. It utilizes identical inputs and deletes the file synchronously. A try-catch block is used to handle errors, and console.error() is used to log errors. Console.log() is used to record a successful deletion. The specified file will be eliminated from the file system after executing this code. Ensure that the location of the file you want to remove is substituted for "path/to/file.txt".
Conclusion
File handling in Node.js is an essential component of development since it makes it possible to manipulate files effectively. A complete collection of functions to read, write, update, and delete files are offered by the fs module. Developers can ensure non-blocking I/O and enhance application performance by utilizing asynchronous operations. For reliable file management, proper error handling is essential. Reliability and security are improved by adhering to best practices such as validating file paths, controlling permissions, and performance optimization. Developers may do a range of file-related operations with Node.js, from managing user uploads to working with configurations. Developers may create sophisticated applications with seamless file manipulation capabilities thanks to Node.js' effective file management.
FAQs
Q. Can I read a file in Node.js synchronously?
A. Yes, you can read a file synchronously in Node.js using the fs.readFileSync method. However, it's generally recommended to use asynchronous file reading (fs.readFile) to avoid blocking the execution of other code.
Q. How can I handle errors while performing file operations in Node.js?
A. Error handling is essential in file operations. You can handle errors using try-catch blocks for synchronous operations or by providing a callback function that captures errors for asynchronous operations. Additionally, you can listen for error events emitted by file streams.
Q. How can I delete a file in Node.js?
A. You can delete a file in Node.js using the fs.unlink method for asynchronous deletion or fs.unlinkSync for synchronous deletion. Both methods require the file path as a parameter.
Q. What precautions should I take while handling files in Node.js?
A. When handling files, it's important to validate file paths to avoid security vulnerabilities. Ensure that you have proper file permissions to perform the desired operations. Additionally, consider using asynchronous operations to avoid blocking the event loop and optimize performance.
Q. Can I update a specific portion of a file in Node.js?
A. Yes, you can update specific portions of a file in Node.js. However, you would need to read the file, make the necessary modifications to the data, and then write the updated data back to the file.