A software design pattern called CQRS (Command Query Responsibility Segregation) divides up who is responsible for accessing and writing data in a system. The same set of components are frequently used in traditional design patterns for both reading and writing data. CQRS introduces two distinct models to suggest the division of these duties.
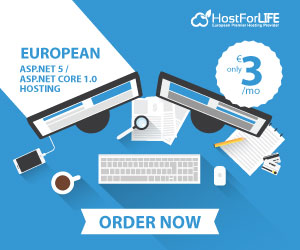
- Command Model: The command model handles event triggering, data store updating, and command processing and validation.
- Query Model: This model manages the processes involved in getting data out of the system.
The four major concepts of CQRS are listed below:
- Command: Action or request to modify the system's status
- Query: An occurrence or a request to obtain data from the system
- Command Handler: An element in charge of processing commands and adjusting the system's state.
- Query Handler: An element in charge of managing queries and getting information out of the system.
CQRS Offers Advantages like:
- Scalability: Because the application supports several read and write data models, it can be scaled.
- Flexibility: It permits the use of distinct data stores that are best suited for writing and reading processes.
- Performance: There is room for improvement with this application.
This CQRS Model may be used with Nest JS; in this tutorial, we'll discover how to do it.
We'll use the creation and retrieval of a TODO application as an example using the CQRS paradigm.
Application for TODOs using CQRS pattern
You must use the command line to install nest cli if it is not already installed globally on your computer.
npm i -g @nestjs/cli
Create new Nest Js project if not created already using below command
nest new todo-application
Dependency Installation
Nest JS provides a package to implement CQRS, we need to install the package first using below command
npm install --save @nestjs/cqrs
Module Creation
Create new Module for our TODO application using command
nest generate module todo
Commands
Commands are used to change the application state, when a command is triggered, it is handled by corresponding command handler. Then handler will be responsible to process the operation. Every command will have command handler in order to process the command
Create new file inside todo module with name create-todo-command.ts and implement the below code
import { ICommand } from '@nestjs/cqrs';
export class CreateToDoCommand implements ICommand {
constructor(
public readonly title: string,
public readonly description: string,
) {}
}
Command Handler
Create new file inside todo module with name create-todo-command-handler.ts and implement the below code
import { CommandHandler, ICommandHandler } from '@nestjs/cqrs';
import { CreateToDoCommand } from './create-todo-command';
@CommandHandler(CreateToDoCommand)
export class CreateToDoHandler implements ICommandHandler<CreateToDoCommand> {
async execute(command: CreateToDoCommand): Promise<void> {
// Add Logic to do validation and business rule
const { title, description } = command;
// Use Repository to save directly or Create Factory to add business logic and save
}
}
Query
Queries are used to retrieve the data from the application, when a query is requested, Query handler handles the requests and retrieves the data. Every query will have query handler
Create new file inside todo module with name get-todo-query.ts and implement the below code
import { IQuery } from '@nestjs/cqrs';
export class GetToDoQuery implements IQuery {
constructor() {}
}
Query Handler
Create new file inside todo module with name get-todo-query-handler.ts and implement the below code
import { IQueryHandler, QueryHandler } from '@nestjs/cqrs';
import { GetToDoQuery } from './get-todo-query';
@QueryHandler(GetToDoQuery)
export class GetToDoQueryHandler implements IQueryHandler<GetToDoQuery> {
async execute(query: GetToDoQuery): Promise<any> {
// Fetch data using repository or factory and return it
// Sample Response
return [
{ id: 1, title: 'Test', description: 'Reminder to complete daily activity' },
{ id: 2, title: 'Test 2', description: 'Reminder to complete daily activity2' },
];
}
}
Module
In the todo.module.ts file, import the CQRS module to use the command handlers and query handlers
import { Module } from '@nestjs/common';
import { CqrsModule } from '@nestjs/cqrs';
import { ToDoController } from './todo.controller';
import { CreateToDoHandler } from './create-todo-command-handler';
import { GetToDoQueryHandler } from './get-todo-query-handler';
@Module({
imports: [CqrsModule],
controllers: [ToDoController],
providers: [CreateToDoHandler, GetToDoQueryHandler],
})
export class ToDoModule {}
Controller
Create todo.controller.ts file to handle the API request and use command and query bus
import { Controller, Get, Post, Body } from '@nestjs/common';
import { CommandBus, QueryBus } from '@nestjs/cqrs';
import { CreateToDoCommand } from './create-todo-command';
import { GetToDoQuery } from './get-todo-query';
@Controller('ToDo')
export class ToDoController {
constructor(
private readonly commandBus: CommandBus,
private readonly queryBus: QueryBus,
) {}
@Post()
async createToDo(@Body() body: { title: string, description: string }): Promise<void> {
const { title, description } = body;
await this.commandBus.execute(new CreateToDoCommand(title, description));
}
@Get()
async getToDo(): Promise<any[]> {
return this.queryBus.execute(new GetToDoQuery());
}
}
Pro Tips
You can create a separate folder for command and query to segregate the code and make it more readable. Also, here I just gave the basic high level demo, but in your application, you can create dto for command and query, and you can directly use that dto
Conclusion
In conclusion, while CQRS can provide advantages in terms of scalability and flexibility, it comes with increased complexity and potential challenges in terms of consistency and development overhead. It is important to carefully consider whether the benefits align with the specific requirements and goals of the application being developed.
HostForLIFE.eu Node.js Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
