
October 16, 2020 07:42 by
Peter
Binding is basically the process of connecting data between the view of your application and it's code behind.
In Angular, the view of the application is the HTML page and the code behind is the Component class written in typescript code.
There are different types of data binding in Angular,
Component to View using interpolation
This is one of the ways of bindings provided by the Angular framework. For this one, we need to have a class level property in our Component class which we use in our HTML using double curly braces.
For example, the below code snippet shows a piece of code in the component class. There are 3 properties: department, imgURL and
showspinner, out of which imgURL and showspinner are already initialized, whereas department is just declared.
department: Any;
imgURL: string = "assets/photos/Department.jpg";
showSpinner: boolean = false;
In our HTML file, these properties are used inside double curly braces to render these values directly on the browser. In our case, the imgURL represents the source of the image so that has to be used in the below manner, as shown in the below code snippet.
<image src = {{imgURL}} >
When the application gets rendered on the browser, {{imgURL}} gets replaced by assets/photos/Department.jpg.
In the real time application, this property is initialized dynamically at runtime.
Component to View using property binding
Just like interpolation, this is another type of one way binding. Just like the prior one, in property binding also, we need a class level property that has to be bound with an HTML control on the View. However, the syntax is a little different.
Let's use the same example to apply to property binding.
department: Any;
imgURL: string = "assets/photos/Department.jpg";
showSpinner: boolean = false;
To use the property binding we need to use the below syntax. We need to enclose the property of the HTML control inside the square braces and enclose the Component property inside the quotes.
<image [src] = 'imgURL' >
Note
While rendering the data on UI, interpolation converts the data into string, whereas property binding does not change the type and renders it as it is.
View to Component using event binding
This type of binding is used to bind data from View to Component i.e. from the HTML page to the Component class. This one is similar to the events of simple javascript. It can either be a simple click event, a keyup, or any other. The only difference is that the events in Angular have to be put inside circular braces, the rest all is same.
As shown in the below code snippet, there are 3 buttons with their respective click events. The methods handling those events are in the code behind file.
<button (click) = 'addDepartment()' >Add </button>
<button (click) = 'editDepartment()' >Edit </button>
<button (click) = 'deleteDepartment()' >Delete </button>
To Bind View and Component Simultaneously (two-way binding)
This type of binding is a little different from other frameworks. The two-way binding keeps the property in the Component class and the value of the HTML control in sync. Whenever we change the value of HTML control, the value of the property of the Component class also
changes.
To implement this type of binding in Angular, we use a special directive with a little bit different of a syntax.
<input required [(ngModel)] = 'departmentName' name = 'departmentName' >
As you can see in the above code snippet, a directive ngModel has been used inside 2 types of braces. The two braces signify two different bindings. The square brace is for property binding that we discussed as the second type and the circular is for event binding, the third one
that we discussed.
Lets talk a little about this textbox, whenever there is any change in the value of this textbox an event gets triggered, and by means of the event binding the value gets passed to the Component property and it gets updated and similarly whenever there is any change in the property value, the value of the textbox will also get updated by means of property binding.
The Component property associated with this textbox in the above code is departmentName.
Take an example, when we are fetching some data by making an API call, at the beginning the textbox won't have any value but as soon as the value of the property in the Component class gets updated, the value of textbox will also get updated simultaneously.
Note
In order to use [(ngModel)] for two-way binding, the name attribute is a must. The Angular framework internally uses the name attribute to map the value of HTML control with the Component property.
HostForLIFE.eu AngularJS Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
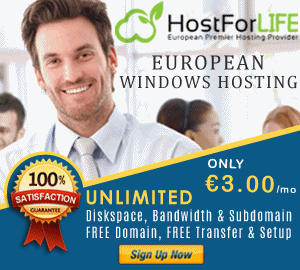

October 15, 2020 10:08 by
Peter
In this blog, we will be talking about how transactions take place in Entity Framework. DbContext.Database.BeginTransaction() method creates a new transaction for the underlying database and allows us to commit or roll back changes made to the database using multiple SaveChanges method calls.
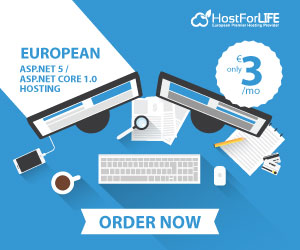
The following example demonstrates creating a new transaction object using BeginTransaction(), which is, then, used with multiple SaveChanges() calls.
using(var context = new SchoolContext()) {
using(DbContextTransaction transaction = context.Database.BeginTransaction()) {
try {
var standard = context.Standards.Add(new Standard() {
StandardName = "1st Grade"
});
context.Students.Add(new Student() {
FirstName = "Rama2",
StandardId = standard.StandardId
});
context.SaveChanges();
context.Courses.Add(new Course() {
CourseName = "Computer Science"
});
context.SaveChanges();
transaction.Commit(); //save the changes
} catch (Exception ex) {
transaction.Rollback(); //rollback the changes on exception
Console.WriteLine("Error occurred.");
}
}
}
In the above example, we created new entities - Standard, Student, and Course and saved these to the database by calling two SaveChanges(), which execute INSERT commands within one transaction.
If an exception occurs, then the whole changes made to the database will be rolled back.
I hope it's helpful.