The use of "pipes" is one of Angular's most potent tools for formatting and changing data inside templates. Developers can apply transformations like formatting dates, changing text cases, or even filtering data in an efficient and reusable way by using pipes, which offer a declarative mechanism to handle data before it is shown to the user.
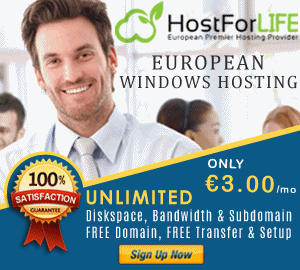
Writing clean, manageable, and modular code for Angular applications requires an understanding of pipes. The main distinctions between pipes and functions will be discussed in this post, along with how to use built-in pipes and make your own custom pipes to increase Angular's functionality. You will have a firm grasp on how to integrate pipes into your Angular projects to improve user experience and expedite data presentation by the end of this tutorial.
What is an Angular Pipe?
In Angular, a pipe is a way to transform data before it is displayed in the user interface. Pipes can be used in templates to modify or format data without having to alter the original data.
Pipes are an Angular concept, not a TypeScript (TS) feature. They are a core part of Angular’s template syntax and are used to transform data in the view (template) layer of Angular applications.
Key Points about Pipes in Angular
- Angular-Specific: Pipes are a built-in feature of the Angular framework designed to be used in Angular templates. They are not a native feature of JavaScript or TypeScript.
- Purpose: Their primary function is to transform data in the template before it is displayed to the user. This transformation can include formatting dates, numbers, currencies, filtering arrays, or performing more complex data transformations.
Declarative Transformation: Pipes enable declarative transformation of data within the template, meaning that the logic for transforming data is cleanly abstracted away from the component’s TypeScript code.
You may be wondering why we should use Pipes when we can use functions.
Criteria |
Pipe |
Function |
Purpose |
Data transformation in the template |
Business logic and calculations |
Use case |
Formatting, filtering, sorting, etc. |
Complex or multi-step calculations |
Performance |
Pure pipes are efficient for transforming data only when needed |
Functions can be less performant when used in templates (requires manual calls) |
Reusability |
Highly reusable across templates |
Functions are reusable within the component or service |
Asynchronous Handling |
Handles observables and promises with AsyncPipe |
Requires manual subscription logic or use of 'async' in templates |
Complexity |
Best for simple, declarative transformations |
Best for complex or dynamic logic |
When to use |
When transforming data for display in the template |
When performing business logic or side effects that don't belong in the template |
Types of Pipes
There are two types of Pipes.
Pure Pipe (Default): A pure pipe will only re-run when its input value changes.
@Pipe({
name: 'pureExample',
pure: true // This is the default value, so you can omit this
})
export class PureExamplePipe implements PipeTransform {
transform(value: any): any {
console.log('Pure pipe executed');
return value;
}
}
Impure Pipe: An impure pipe will re-run whenever Angular detects a change in the component’s state, even if the input value hasn’t changed.
@Pipe({
name: 'impureExample',
pure: false // Set to false to make it impure
})
export class ImpureExamplePipe implements PipeTransform {
transform(value: any): any {
console.log('Impure pipe executed');
return value;
}
}
In Angular, you can use in-built pipes or create your own.
In-built pipes
Angular provides some basic pipes that can be used.
It comes from the '@angular/common' package.
Some popular ones that can be helpful are:
CurrencyPipe, DatePipe, DecimalPipe, LowerCasePipe, UpperCasePipe and TitleCasePipe
How to use an in-built pipe?
In your ts file, define your variable. In our example, we will use the variable title.
title = 'app works!';
In your html, you can use the pipe as follows:
<h1> {{title | uppercase}} </h1>
The result is how the string title is displayed:

Note. The currency ‘USD’ is added in front because of the currency pipe, and only 10 characters are displayed because of the slide pipe.
Custom pipes
Run the command below to create a pipe file:
ng generate pipe <<pipe-name>>.
For example: ng generate pipe my-custom-pipe. Once executed, the two files below will be created

Open the file ‘my-custom-pipe.pipe.ts. You will see the following boilerplate code provided:
import { Pipe, PipeTransform } from '@angular/core';
@Pipe({
name: 'myCustomPipe'
})
export class MyCustomPipePipe implements PipeTransform {
transform(value: any, args?: any): any {
return null;
}
}
After the default class, you can create the function for your new pipe. In our case, we will create a pipe that will replace spaces in a hyphen. It is important to add the decorator ‘@Pipe’ before the class so that Angular knows what follows will be a pipe. Also, pass the name of the pipe as a parameter in the ‘@Pipe’ decorator. Also, when creating the class, implement ‘PipeTransform’. The resulting class will be as follows:
@Pipe({name: 'removeWhiteSpace'})
export class RemoveWhiteSpacePipe implements PipeTransform {
transform(value: string): string {
return value.replace(/\s+/g, '-');
}
}
The resulting class will be as follows (the full code):
import { Pipe, PipeTransform } from '@angular/core';
@Pipe({
name: 'myCustomPipe'
})
export class MyCustomPipePipe implements PipeTransform {
transform(value: any, args?: any): any {
return null;
}
}
@Pipe({name: 'removeWhiteSpace'})
export class RemoveWhiteSpacePipe implements PipeTransform {
transform(value: string): string {
return value.replace(/\s+/g, '-');
}
}
In the ts file of your component, create the variable that will hold the value that will be transformed
textWithSpaces = 'This is a text with a lot of spaces that will be transformed';
In the html file of your component, do the following:
<p>{{ textWithSpaces | removeWhiteSpace }}</p>
The result is the following:

Conclusion
An effective and potent method for formatting and transforming data in the templates of your application is to use angular pipes. Without having to code repetitious logic in your components, you can quickly manipulate data types like strings, dates, and numbers by utilizing built-in pipes. More freedom is provided by custom pipelines, which let you design modular, reusable, and maintainable transformation logic that is suited to your particular requirements.
Understanding the distinction between pipes and functions is key to leveraging their full potential. While functions provide a direct way to execute code, pipes offer a declarative approach to handle transformations directly within templates, improving readability and performance.
Whether you're working with Angular’s built-in pipes or creating custom ones, the ability to easily manipulate data in the view layer is a significant advantage in building dynamic and user-friendly applications. By mastering Angular pipes, you can keep your code clean, concise, and aligned with best practices, ultimately leading to more maintainable and scalable applications.
With the knowledge of how to use and create pipes, you’re now equipped to enhance your Angular applications with powerful data transformations, making your development experience more efficient and enjoyable.