
February 23, 2017 08:24 by
Peter
A message exchange pattern means how the client and WCF service exchange the message (data) internally. There are a total three types of patterns,
- Request – Reply
- One Way
- Duplex
We will learn each of them in detail below.
Request – Reply
In request reply pattern client sends the request to WCF service and waits for the response from the WCF service. Until they get a reply no other process runs. After getting a response from the WCF service they will continue its work. If WCF service has a method with void return type then the client will wait for the reply.
So in this pattern if any error occurs then it will directly display on the client side. Default pattern for any methods is request – reply. You can declare any method to request a reply by assigning ‘IsOneWay=false’ as show below.
[ServiceContract]
public interface IMessageExchPatterns
{
[OperationContract]
string GetDataRequestReply (string name);
}
OR
[ServiceContract]
public interface IMessageExchPatterns
{
[OperationContract(IsOneWay=false)]
string GetDataRequestReply (string name);
}
As shown in the above example you can declare request reply pattern in two ways.
One Way
In One way pattern client sends the data or request to WCF service but it doesn't wait for any reply from the service and also service doesn't send any reply to them, so here the client will not show any thing on service then will only send the message to service. It doesn't check if that message reaches the service or not. You can set this pattern by IsOneway=true.
Example
[ServiceContract]
public interface IMessageExchPatterns
{
[OperationContract(IsOneWay=true)]
void GetDataOneWay (string name)
}
Duplex
In Duplex pattern you have to predict that there are two clients (client-1, Client-2); client-1 makes request to service and then service will sent request to client-2 and then service gets response from client-2 and give this replay to client-1.
Here this pattern is to use the callback method for the internal (client to client ) communication. So here we have to define the callback interface as show below,
[ServiceContract(CallbackContract=typeof(IMessageExchPatternsCallback))]
public interface IMessageExchPatterns
{
[OperationContract(IsOneWay = false)]
string GetDataRequestReply(string name);
[OperationContract(IsOneWay=true)]
void GetDataOneWay(string name);
[OperationContract]
void GetDataDuplex(string name);
}
public interface IMessageExchPatternsCallback
{
[OperationContract]
void SendData(string name);
}
As shown in above example we specify the,
[ServiceContract(CallbackContract=typeof(IMessageExchPatternsCallback))]
Here we specify the call back contract for the interface. This callback interface (IMessageExchPatternsCallback) is implementing on client side.
[ServiceBehavior(ConcurrencyMode = ConcurrencyMode.Reentrant)]
public class MessageExchPatterns : IMessageExchPatterns
{
public string GetDataRequestReply(string name)
{
return "You have enter : " + name;
}
public void GetDataOneWay(string name)
{
Console.Write("You have enter : " + name);
}
public void GetDataDuplex(string name)
{
OperationContext.Current.GetCallbackChannel<IMessageExchPatternsCallback>().SendData("Hi, " + name);
}
}
Now host this service on console application
ServiceHost host = new ServiceHost(typeof(MessageExchPatterns.MessageExchPatterns));
host.Open();
Console.Write("Service started...");
Console.Read();
Now create client application with Window form application as below.
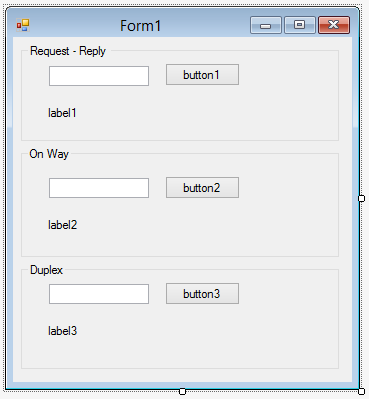
Write code as shown below,
[CallbackBehavior(UseSynchronizationContext = false)]
public partial class Form1 : Form,ServiceReference1.IMessageExchPatternsCallback
{
public Form1()
{
InitializeComponent();
}
InstanceContext instanceContext;
private void button1_Click(object sender, EventArgs e)
{
ServiceReference1.MessageExchPatternsClient clnt = new ServiceReference1.MessageExchPatternsClient(instanceContext);
label1.Text = clnt.GetDataRequestReply(textBox1.Text);
}
void ServiceReference1.IMessageExchPatternsCallback.SendData(string name)
{
Label3.Text = name;
}
private void button2_Click(object sender, EventArgs e)
{
MessageExchPatternsClient clnt = new MessageExchPatternsClient(instanceContext);
clnt.GetDataOneWay(textBox2.Text);
}
private void button3_Click(object sender, EventArgs e)
{
ServiceReference1.MessageExchPatternsClient clnt = new ServiceReference1.MessageExchPatternsClient(instanceContext);
clnt.GetDataDuplex(textBox3.Text);
}
private void Form1_Load(object sender, EventArgs e)
{
instanceContext = new InstanceContext(this);
}
}
Now run the WCF service host. Then run client application.
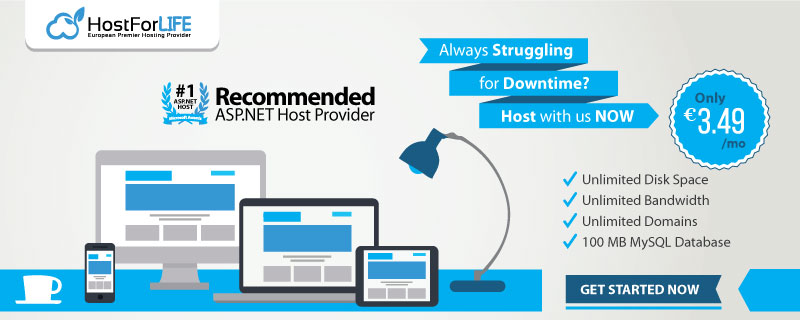