Top Links navigation is one of the basic blocks in Magento. Top links block allows you to create a personal area for the customer within your online store. Top Links includes: Login/Logout, My Account, My Wishlist, My Cart and Checkout links. By default «Top links» are located in the header, but they can be moved wherever you want if it’s needed. In this tutorial, we will go through how to manage and improve Top Links in Magento.
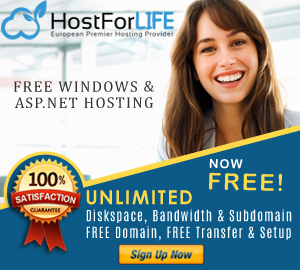
One of the most important differences between top links and regular static links is that when you add products to the cart or to your wishlist, top links automatically records products which were added.
Example of Top links in the default Magento theme in the header:

Usage of Top links in Magento
At first we have to call the block.
php echo this->getChildHtml(‘topLinks’) ?>
You may not create in template: template/page/html/header.phtml, but creates in page.xml
<block type=”page/html_header” name=”header” as=”header”>
<block type=”page/template_links” name=”top.links” as=”topLinks”/>
<block type=”core/text_list” name=”top.menu” as=”topMenu”/>
</ block>
Now we need to add links to this block by using the command:
<action method=”addLink” translate=”label title” >…</action>
We gotta make it in the following XML files:
- Login/Logout, My Account – customer.xml
- My Cart and Checkout – checkout.xml
- My Wishlist – wishlist.xml
It should be noted that link to My Cart calls by the command:
<action method=”addCartLink”></action>
and
<action method=”addCheckoutLink”></action>
for Checkout link.
How to edit Top Links in Magento
All top links are based on a template which is located here: page/template/links.phtml. Here you can add additional classes or commit needed changes.
Often people want to use separate links. For example Login/Logout and My Account should be on the left side and My Wishlist, My Cart and Checkout on the right side.
Something like on the example below:
We can make this in a few simple steps as below:
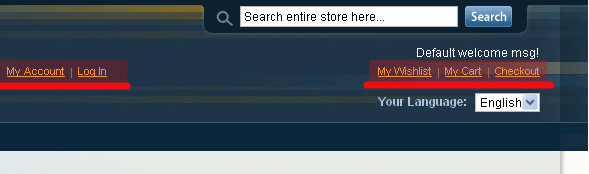
Open page.xml and create another block there, almost identical to “topLinks” but with name “topLinksLeft”;
<block type=”page/html_header” name=”header” as=”header”>
<block type=”page/switch” name=”store_language” as=”store_language” template=”page/switch/languages.phtml”/>
<block type=”core/text_list” name=”top.menu” as=”topMenu”/>
<block type=”page/template_links” name=”top.links” as=”topLinks”/>
topLinksLeft”/>
</ block>
In template template/page/html/header.phtml with help of the command:
php echo this->getChildHtml(‘topLinksLeft’) ?>
We can call our block in the proper place:
<div>
<h1 id=”logo” title=”<?php echo $this->getLogoAlt() ?>” style=”background-image:url(<?php echo $this->getLogoSrc() ?>);”><a href=”<?php echo $this->getUrl(”) ?>”><?php echo $this->getLogoAlt() ?></a></h1>
<div><?php echo $this->getChildHtml(‘topLinksLeft’) ?></div>
<?php echo $this->getChildHtml(‘topLinks’) ?>
<?php echo $this->getChildHtml(‘topMenu’) ?>
</div>
When you’ve done, open customer.xml where we have to change the name of the block which is responsible for Login/Logout, My Account. We are changing its name from “top.links” on “top.links.left” as in example:
customer_logged_in>
<reference name=”top.links.left”>
<action method=”addLink” translate=”label title” module=”customer”><label>My Account</label><url helper=”customer/getAccountUrl”/><title>My Account</title><prepare/><urlParams/><position>10</position></action>
</reference>
<reference name=”top.links.left”>
<action method=”addLink” translate=”label title” module=”customer”><label>Log Out</label><url helper=”customer/getLogoutUrl”/><title>Log Out</title><prepare/><urlParams/><position>100</position></action>
</reference>
</customer_logged_in>
We also can assign other template to the links on the left ( rather useful in some cases) For that we just have to duplicate template “page/template/links.phtml” and call it links_left.phtml. So now we have 2 templates “links.phtml” for the right side and “links_left.phtml” for the left side. Now all we need to do is just connect it. For connection we use block «topLinksLeft» page.xml and change it to links_left.phtml.
<block type=”page/html_header” name=”header” as=”header”>
<block type=”page/template_links” name=”top.links” as=”topLinks”/>
<block type=”page/template_links” name=”top.links.left” as=”topLinksLeft” template=”page/template/links_left.phtml” />
</ block>
Now you can apply different styles and HTML for the left and the right side.
Wow, almost forgot about “Register” button which is usually located near the “Login/Logout” button. No worries about that as well. As you can already guess we start from customer. xml file where we do next, if we want to add “Register” button to the top links:
<customer_logged_out>
<reference name=”top.links”>
<action method=”addLink” translate=”label title” module=”customer”> <label> Log In </ label> <url helper=”customer/getLoginUrl”/> <title> Log In </ title> <prepare /> <urlParams/> <position> 100 </ position> </ action>
<action method=”addLink” translate=”label title” module=”customer”> <label> register </ label> <url helper=”customer/getRegisterUrl”/><title>register</title><prepare/><urlParams/><position>10</position></action>
</ reference>
</ customer_logged_out>
Happy Coding! Hope it works for you.
Magento with Free ASP.NET Hosting
Try our Magento with Free ASP.NET Hosting today and your account will be setup soon! You can also take advantage of our Windows & ASP.NET Hosting support with Unlimited Domain, Unlimited Bandwidth, Unlimited Disk Space, etc.
