In this post, I'll show you how to increase your productivity using.NET Core CLI Commands and how to use.NET Core CLI (Command Line Interface) commands to assist developers speed up their work with a few tips and tricks. We can create, build, add packages, restore packages, run apps, and deploy Core applications using the.Net CLI. I'll go over basic operations like establishing new projects, compiling and running programs, managing dependencies, and working with NuGet packages.
Increasing Productivity with the Command Line Interface in Visual Studio
When we develop a new application in Visual Studio, we use.Net CLI commands internally to create, build, add packages, launch, and deploy apps.
When you install the.NET Core SDK, the.NET Core CLI is also installed by default. As a result, no additional installation is required.CLI for Net Core.
How can I tell if.Net CLI is installed or not?
Open Run and type CMD.
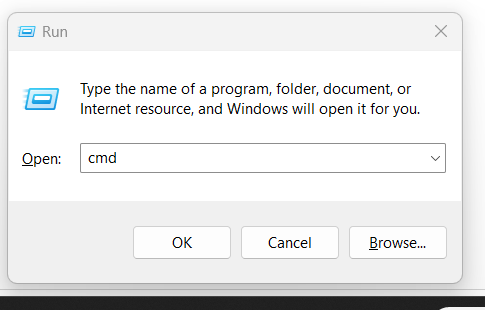
When the command prompt appears, type dotnet and press enter.
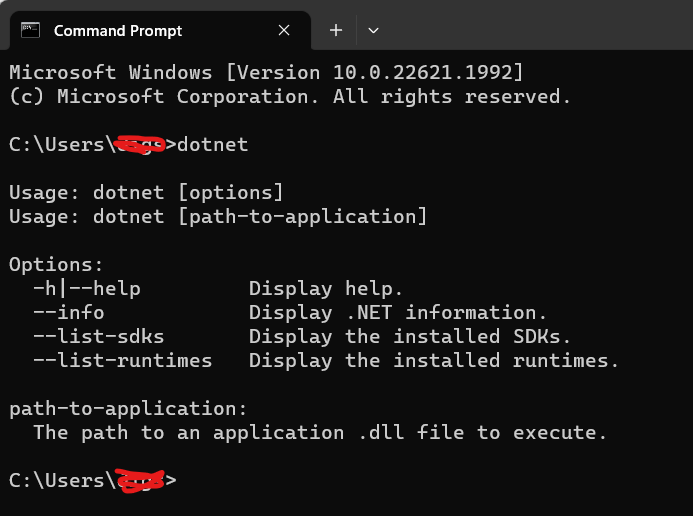
CLI Command Syntax: dotnet command> argument> option>
Take note that all commands begin with dotnet. The syntax shown above will assist developers in executing essential instructions from the command-line interface. Let's use the first command (dotnet help) to get help for all commands.
How do I obtain a complete list of all.Net Core commands?
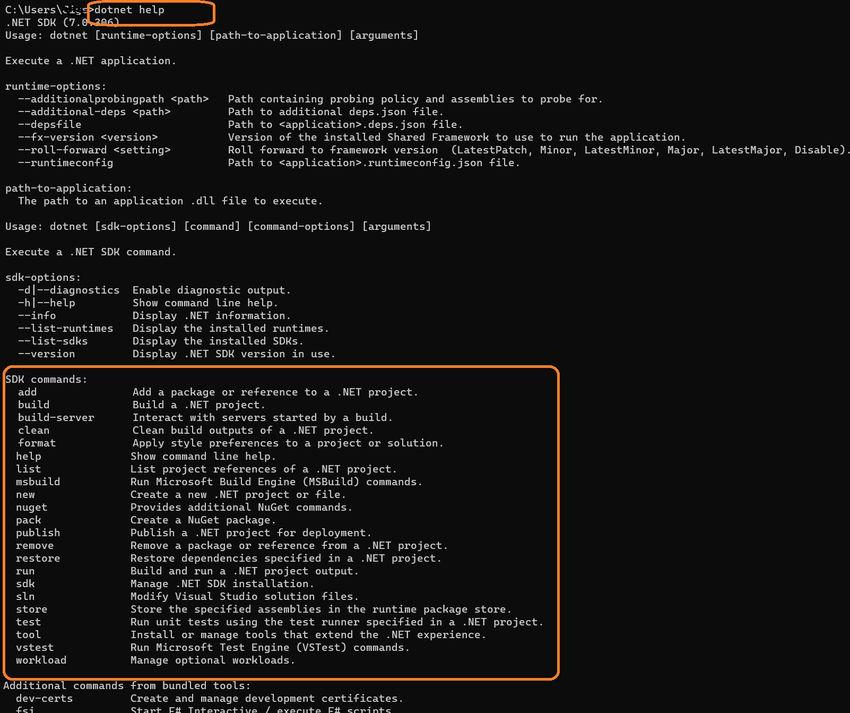
List of all .Net Core commands
Below is a list of all .Net core commands that will appear once we run the "dotnet help" command. Let's list down all commands as below with a short description of what each does.
SDK commands
- add: Add a package or reference to a .NET project
- build: Build a .NET project
- build-server: Interact with servers started by a build
- clean: Clean build outputs of a .NET project
- format: Apply style preferences to a project or solution
- help: Show command line help
- list: List project references of a .NET project
- msbuild: Run Microsoft Build Engine (MSBuild) commands.
- new: Create a new .NET project or file.
- NuGet: Provides additional NuGet commands.
- pack: Create a NuGet package.
- publish: Publish a .NET project for deployment
- remove: Remove a package or reference from a .NET project.
- restore: Restore dependencies specified in a .NET project.
- run: Build and run a .NET project output.
- SDK: Manage .NET SDK installation.
- sln: Modify Visual Studio solution files.
- store: Store the specified assemblies in the runtime package store.
- test: Run unit tests using the test runner specified in a .NET project.
- tool: Install or manage tools that extend the .NET experience.
- vstest: Run Microsoft Test Engine (VSTest) commands.
- workload: Manage optional workloads.
How to create a new console project?
Let's create a new console project using the "dotnet new" Command.
dotnet new console -n MyConsoleAppUsingCLI -o C:\Users\XXXXX\projects\MyConsoleAppUsingCli
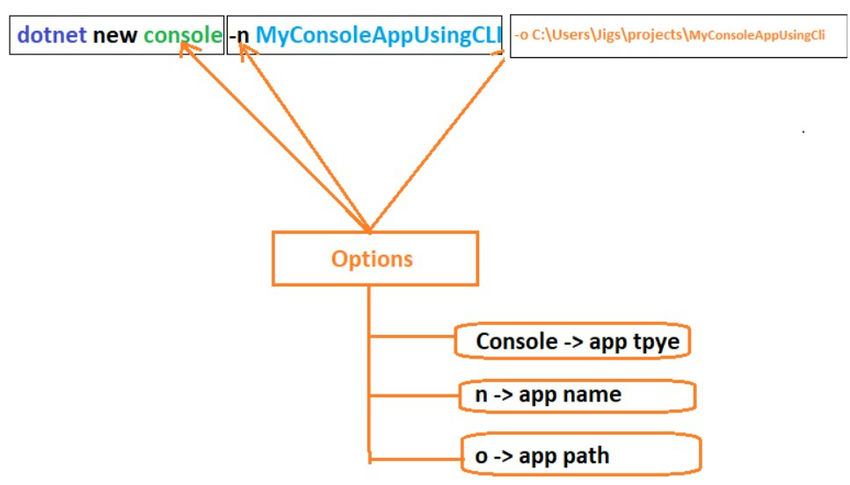
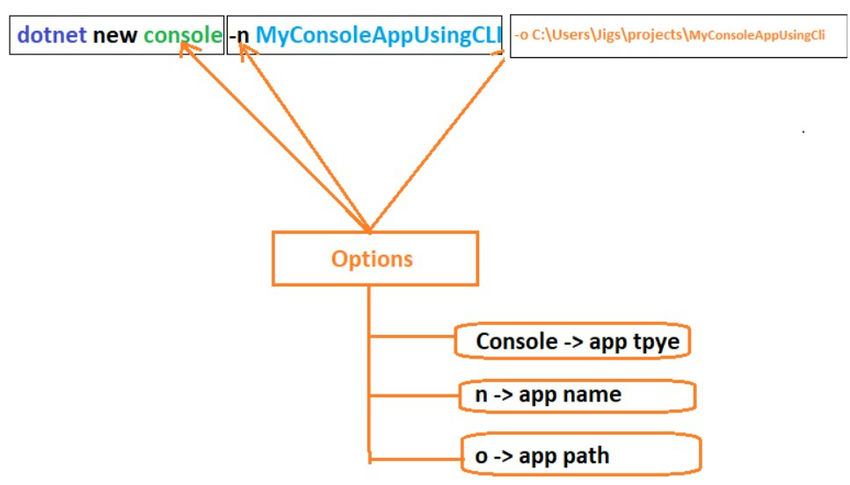
Once the above Command runs successfully, We are able to create a console application with the name "MyConsoleAppUsingCli" at the given path "C:\Users\XXXXX\projects\". Let's build the newly created project using the build command.
Build Command: dotnet build C:\Users\peter\projects\MyConsoleAppUsingCli\
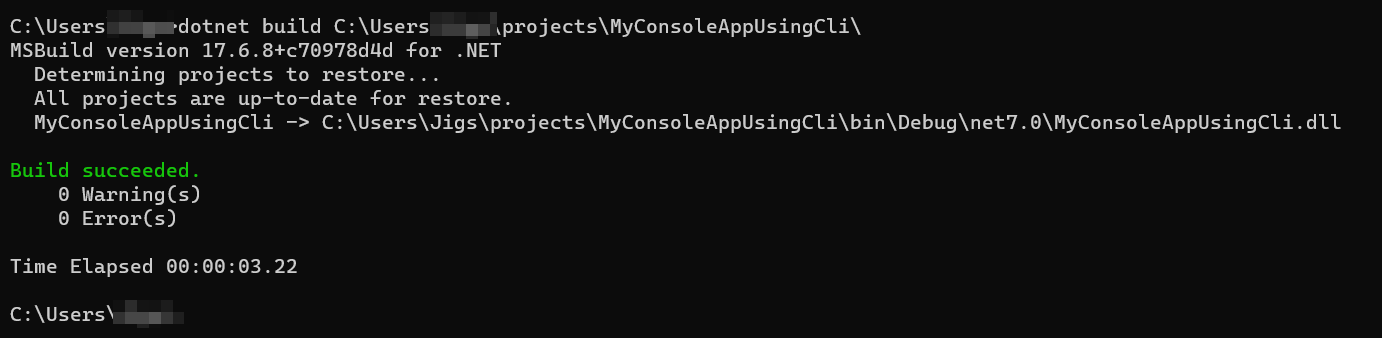
Once we run the build command, As per the above screen, the project build succeeded.
How to add a package reference to the new console application?
Let's add a package reference to the new console application using the add package CLI command.
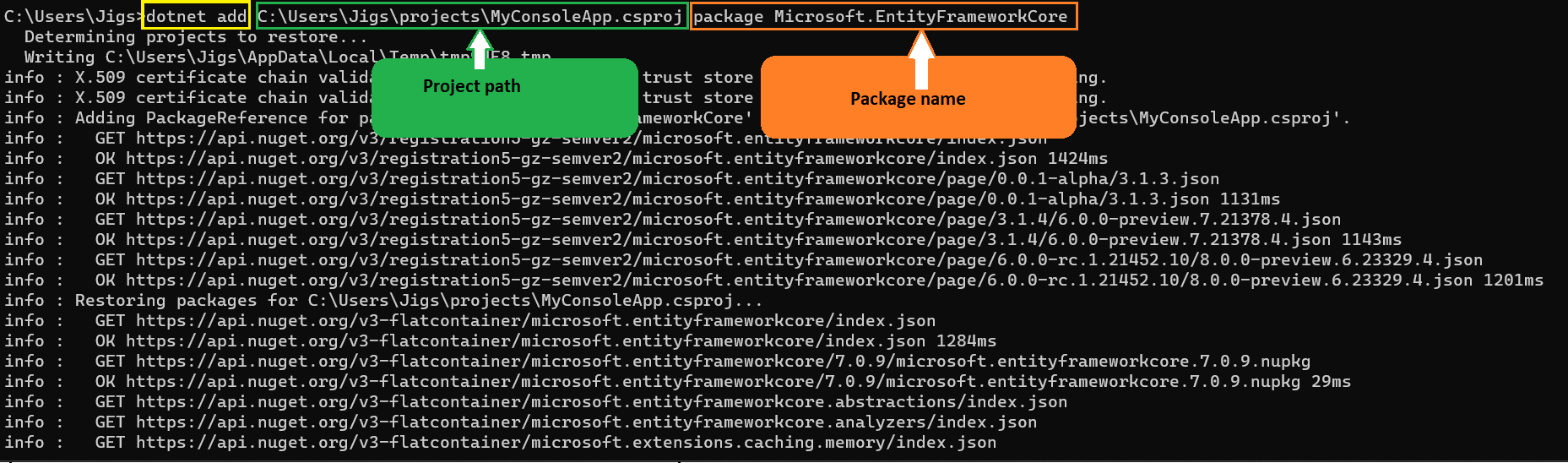
We have added the package "Microsoft.EntityFrameworkCore" to our project. Let's open our console app in Visual Studio and check the newly added package reference in the solution.
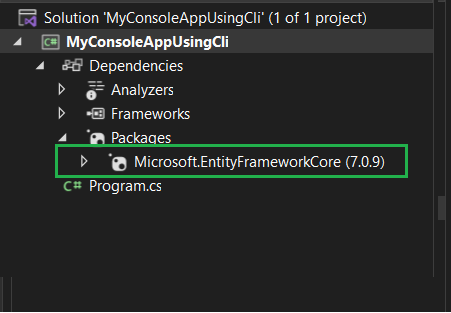
As per the above screen, We are able to see Microsoft.EntityFrameworkCore package reference was added successfully.
Run the project using Run CLI Command
Run project using Run CLI Command: dotnet run --project C:\Users\peter\projects\MyConsoleAppUsingCli\MyConsoleAppUsingCli.csproj
In this article, I have demonstrated how to boost productivity with Visual Studio's command-line interface. CLI is very powerful and is used for developing, running, and publishing applications. We have covered essential commands like creating new projects, compiling and running applications, managing dependencies, and handling NuGet packages in console applications. Here I have explained the basic commands required to set up a project and run the new project, but you can explore more on other commands and leverage the power of the.NET Core CLI for streamlined and productive development projects.