
September 6, 2023 09:48 by
Peter
Wrangling application state is a vital skill in the dynamic arena of web development. Keeping code tidy and predictable can be difficult, but the introduction of tools like Immer has provided a breath of fresh air in this sector. Immer has won the hearts of developers all over the world with its ease of use and efficiency in dealing with state changes. This essay will take you on a journey into the world of Immer, looking into its practical implementation, benefits, and how it makes the sometimes difficult chore of state management in JavaScript a lot easier.
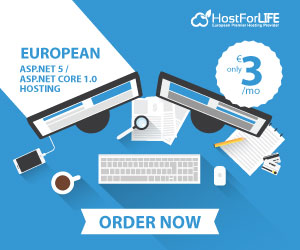
Immer, lovingly created by Michel Weststrate, is a library that changes the way we deal with data immutability. It enables us to write code that appears to modify state directly while actually orchestrating the construction of new, immutable state structures.1. Starting Out: InstallationLet's begin your Immer trip by installing the library with npm or yarn.
npm install immer
2. Basic Usage
At the core of Immer is a function called produce. It's the magic wand that takes your current state and a function containing your desired changes and works its enchantment.
import produce from 'immer';
const initialState = { count: 0 };
const nextState = produce(initialState, draftState => {
draftState.count += 1;
});
3. Taming Nesting
Now comes the pièce de résistance. Immer's prowess shines when dealing with deeply nested objects and arrays. It simplifies complex state structures with ease.
const complexState = {
user: {
name: 'Alice',
address: {
city: 'Wonderland'
}
}
};
const newState = produce(complexState, draftState => {
draftState.user.name = 'Bob';
draftState.user.address.city = 'Dreamland';
});
Benefits
Crystal Clarity: Immer brings lucidity to your code. Instead of fretting about cloning and immutability, you can focus on the changes you wish to make.
Performance Star: The magic of Immer not only simplifies your code but optimizes performance by reducing memory overhead and avoiding redundant copying.
Readable Harmony: Code created with Immer mirrors traditional mutable code, fostering understanding and maintainability.
Conclusion
Immer has reimagined the landscape of state management in JavaScript applications. Its knack for handling immutable updates effortlessly, coupled with an approachable syntax, is nothing short of revolutionary. By streamlining the intricate dance of cloning and immutability checks, Immer reduces error risks and transforms the development experience.
In the ever-evolving world of web development, where efficiency and maintainability are paramount, Immer emerges as a steadfast ally. Whether you're working on a modest project or an intricate application, incorporating Immer into your state management arsenal offers cleaner, bug-free code and boosts developer productivity.
So, as you embark on your coding escapades, remember that Immer isn't just a library; it's a compass that guides you through the labyrinth of state management, making your journey more delightful and your code more enchanting.