
February 28, 2020 11:08 by
Peter
Eager loading is the process whereby a query for one type of entity also loads related entities as part of the query, so that we don't need to execute a separate query for related entities.
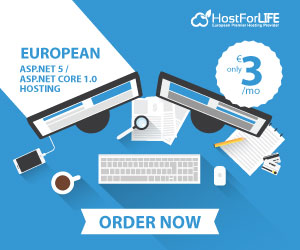
In simple language, Eager loading joins the entities which have foreign relation and returns the data in a single query.
Now, the question is how to properly handle the eager-loading problem for complex object graphs within the Repository pattern.
Let's get started.
Step 1: Add a method into your Interface which eager loads the entities which we specify:
public interface IProjectInterface<T> where T : class
{
Task<IEnumerable<T>> EntityWithEagerLoad(Expression<Func<T, bool>> filter, string[] children);
Task<List<T>> GetModel();
T GetModelById(int modelId);
Task<bool> InsertModel(T model);
Task<bool> UpdateModel(T model);
Task<bool> DeleteModel(int modelId);
void Save();
}
The method EntityWithEagerLoad() takes 2 arguments, one is filter criteria and another is an array of entities which we want to eager load.
Step 2: Define the method EntityWithEagerLoad in your base repository:
public async Task<IEnumerable<T>> EntityWithEagerLoad(Expression<Func<T, bool>> filter, string[] children)
{
try
{
IQueryable<T> query = dbEntity;
foreach (string entity in children)
{
query = query.Include(entity);
}
return await query.Where(filter).ToListAsync();
}
catch(Exception e)
{
throw e;
}
Step 3 : For using this method , call this function from your controller as shown below:
[HttpGet]
[Route("api/Employee/getDetails/{id}")]
public async Task<IEnumerable<string>> GetDetails([FromRoute]int id)
{
try
{
var children = new string[] { "Address", “Education” };
var employeeDetails=await _repository.EntityWithEagerLoad (d => d.employeeId==id,
children);
}
catch(Exception e)
{
Throw e;
}
The variable employeeDetails contains employee details with their Address and education details.

February 14, 2020 08:29 by
Peter
In this article, we'll learn how to use web workers in Angular 8/9.
Web Workers
Web workers speed up in your application when we start working on CPU-intensive tasks. They let you offload work to a background thread. Once we allow a web worker to run, we can use it normally in our application, and the Angular cli will be able to split bundle and code.
The Following Browser versions which are supported in web workers:
- Firefox: 3.5
- Chrome: 4
- Safari: 4
- IE:10
- Opera: 10.6
Installing AngularCLI
Let’s get started by installing Angular CLI, which is the official tool for creating and working with Angular projects. Open a new terminal and run the below command to install Angular CLI.
npm install -g @angular/cli.
If you want to install Angular 9, simply add @next
npm install --g @angular/cli@next
Please check the below command for the Angular version.
ng version
Steps to follow:
Step 1
Create an Angular project setup using the below command or however you want to create it.
ng new projectname
Example,
ng new webworker
Step 2
Now, we must generate web workers from our Angular cli. Open a new terminal and run the below command.
ng generate webWorker my-worker
Create Web Workers In Angular 8/9
Step 3
Now, Create a Web Worker Object in App.component.ts(main scripts)
const worker = new Worker ('./my-worker.worker', {type: 'module'});
We can do some code in App.component.ts (main scripts)
if (typeof Worker !== 'undefined') {
// Create a new
const worker = new Worker('./my-worker.worker', {
type: 'module'
});
worker.onmessage = ({
data
}) => {
console.log(`page got message: ${data}`);
};
worker.postMessage('welcome');
} else {
console.log('Web Workers are not supported in this environment.');
// Web Workers are not supported in this environment.
// You should add a fallback so that your program still executes correctly.
}
Step 4
Now, we can do some code in my-worker.worker.ts
addEventListener('message', ({
data
}) => {
const response = `worker response to ${data}`;
postMessage(response);
});
Web Workers are supported in the browser
Create a new worker (main scripts).
Post message to the worker in my-worker.worker.ts.
When the worker gets the message, addEventListener will fire, and it will create a response to the object then it will call postMessage method.
Step 5
Now, run the application.
npm start
Step 6
Now, we will get the following output,
