
January 16, 2025 07:48 by
Peter
A fundamental element of modern web development is the usage of live chat software to enable real-time user contact. Using technologies like Socket.IO and Node.js greatly simplifies the process. Socket.IO is a JavaScript library that allows bidirectional, real-time communication between web clients and servers, resulting in stable connections across all browsers. Node.js, a stable runtime environment that uses an event-driven, non-blocking I/O model, makes it simple to create scalable network applications.
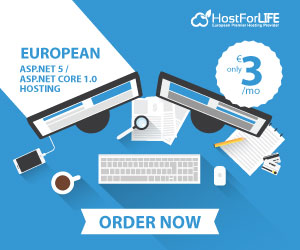
Steps for Creating a Project]
Step 1. Setting Up the Project
Use my previous article for setting up Node.js, "How to upload file in Node.js" In this article, we mentioned important commands for uploading files in Node.js.
Step 2. Setting Up the Server
Create the Server File. Create a file named index.js
const express = require('express');
const app = express();
const { Server } = require('socket.io');
const http = require('http');
const server = http.createServer(app);
const io = new Server(server);
const port = 4000;
app.get('/', (req, res) => {
res.sendFile(__dirname + '/index.html');
});
io.on('connection', (socket) => {
socket.on('send name', (username) => {
io.emit('send name', username);
});
socket.on('send message', (chat) => {
io.emit('send message', chat);
});
});
server.listen(port, () => {
console.log(`Server is listening at the port: ${port}`);
});
Step 3. Creating the Frontend
Create the HTML File. Create a file named index.html
<!DOCTYPE html>
<html>
<head>
<title>Chat app using Socket IO and Node JS</title>
<script src="https://cdn.tailwindcss.com"></script>
<style>
h1 {
color: #273c75;
}
.msg-box {
display: flex;
flex-direction: column;
background: darkgray;
padding: 20px;
}
</style>
</head>
<body>
<h1 class="font-bold text-3xl text-center mt-5">
C# Corner Chat App using Socket IO and Node JS
</h1>
<div>
</div>
<form class="flex flex-col justify-center items-center mt-5" id="form">
<div class="msg-box">
<input class="border border-gray-400 rounded-md mt-5 p-1" type="text" placeholder="Name" id="myname"
autocomplete="off">
<input class="border border-gray-400 rounded-md mt-5 p-1" type="text" placeholder="Message" id="message"
autocomplete="off">
<button class="bg-blue-500 rounded-md p-2 text-white mt-5">
Send
</button>
</div>
</form>
<div class="flex flex-col justify-center items-center mt-5" id="messageArea">
</div>
</body>
<script src="/socket.io/socket.io.js"></script>
<script>
let socket = io();
let form = document.getElementById('form');
let myname = document.getElementById('myname');
let message = document.getElementById('message');
let messageArea = document.getElementById("messageArea");
form.addEventListener("submit", (e) => {
e.preventDefault();
if (message.value) {
socket.emit('send name', myname.value);
socket.emit('send message', message.value);
message.value = "";
}
});
socket.on("send name", (username) => {
let name = document.createElement("p");
name.style.backgroundColor = "#a59494";
name.style.textAlign = "center";
name.style.color = "white";
name.textContent = username + ":";
messageArea.appendChild(name);
});
socket.on("send message", (chat) => {
let chatContent = document.createElement("p");
chatContent.textContent = chat;
messageArea.appendChild(chatContent);
});
</script>
</html>
Step 4. Running the Application
Run the command in CMD.
node index.js
In the output, you can open the chat application in two different browsers or different tabs. When you start typing and send a message, it reflects on both screens in real-time.
Conclusion
You have now successfully used Node.js and Socket.IO to develop a basic live chat application. This application facilitates real-time communication between users by rapidly reflecting messages across several linked clients. To make your app even better, think about including features like private messaging, user authentication, and database storage for conversation history. Using CSS frameworks or original designs to enhance the user interface can improve the user experience. Use Vercel or Heroku to launch your application for increased accessibility. Continue researching and experimenting to create a robust and feature-rich chat experience. Enjoy yourself while coding!