When it comes to document sharing, Adobe's Portable Document Format (PDF) is critical for maintaining the integrity of text-rich and aesthetically pleasing data. Access to online PDF files often necessitates the use of a certain application. Many prominent digital publications now need PDF files. Many companies utilize PDF files to create expert documentation and invoices. Additionally, developers usually employ PDF document generating libraries to meet specific client requirements. The introduction of contemporary libraries has simplified the process of creating PDFs.
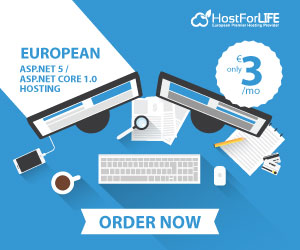
What exactly is Node.js?
Node.js is a cross-platform, open-source server environment that works with Windows, Linux, Unix, macOS, and other operating systems. Node.js is a JavaScript back-end runtime environment that uses the V8 JavaScript engine to execute JavaScript code outside of a web browser.
Developers can utilize JavaScript to construct server-side scripts and command-line tools with Node.js. Dynamic web page content is commonly built before a page is sent to a user's web browser by utilizing the server's ability to run JavaScript code. Node.js promotes a "JavaScript everywhere" paradigm that unifies online application development around a single programming language, as opposed to using several languages for server-side and client-side programming.
const PDFDocument = require('pdfkit');
const fs = require('fs');
const doc = new PDFDocument();
doc.text('Hello world', 100, 100)
doc.end();
doc.pipe(fs.createWriteStream('Output.pdf'));
PDFmake
A wrapper library for PDFKit is called pdfmake . The programming paradigm is where there is the most difference:
While pdfmake uses a declarative approach, pdfkit uses the traditional imperative technique. Because of this, concentrating on what you want to perform is simpler than spending time instructing the library on how to get a particular outcome.
But not everything that glitters is gold, and using Webpack and trying to integrate bespoke fonts may cause problems. Unfortunately, there isn't much information regarding this problem available online. If you don't use Webpack, you can still easily clone the git repository and run the script for embedded font.
var fonts = {
Roboto: {
normal: 'fonts/Roboto-Regular.ttf',
bold: 'fonts/Roboto-Medium.ttf',
italics: 'fonts/Roboto-Italic.ttf',
bolditalics: 'fonts/Roboto-MediumItalic.ttf'
}
};
var PdfPrinter = require('pdfmake');
var printer = new PdfPrinter(fonts);
var fs = require('fs');
var docDefinition = {
// ...
};
var options = {
// ...
}
var pdfDoc = printer.createPdfKitDocument(docDefinition, options);
pdfDoc.pipe(fs.createWriteStream('output.pdf'));
pdfDoc.end();
jsPDF
Among the PDF libraries on GitHub, jsPDF is a PDF generation library for browsers. It has the most starts, and this is not a coincidence given how reliable and well-maintained it is. Because the modules are exported in accordance with the AMD module standard, using them with nodes and browsers is simple.
For PDFKit, the offered APIs follow an imperative paradigm, making it difficult to create complicated layouts. Including typefaces The only additional step is to convert the fonts to TTF files, which is not difficult. Although jsPDF is not the simplest library to use, the extensive documentation ensures that you won't run into any specific difficulties when using it.
import { jsPDF } from "jspdf";
const doc = new jsPDF();
doc.text("Hello world!", 10, 10);
doc.save("output.pdf");
Puppeteer
Puppeteer is a Node library that offers a high-level API to manage Chrome, as you may know, but it can also be used to as PDF generator. Because the templates must be written in HTML, jsPDF is fairly simple for web developers to use.
Puppeteer has mostly two drawbacks. You must put a backend solution in place. Puppeteer must be launched each time a PDF needs to be created, adding to the burden. It moves slowly.
It might be an excellent solution if the aforementioned drawbacks are not a major issue for you, especially if you need to construct HTML tables and other such things.
const puppeteer = require('puppeteer')
async function printPDF() {
const browser = await puppeteer.launch({ headless: true });
const page = await browser.newPage();
await page.goto('www.google.com', {waitUntil: 'networkidle0'});
const pdf = await page.pdf({ format: 'A4' });
await browser.close();
return pdf
})
While pdfmake is based on PDFKit, pdf-lib is a library for producing and editing PDFs that is entirely written in Typescript. Even though it was launched after all the other libraries, it has thousands of stars on GitHub, indicating how well-liked it is.
The APIs have a fantastic design and naturally function with both browsers and nodes.It offers many features that other libraries simply don't have, including PDF merging, splitting, and embedding;
Although it is quite powerful, pdf-lib is also very user-friendly. One of the most popular features is the ability to embed font files using Unit8Array and ArrayBuffer, which enables using fs while dealing with nodes and xhr when working in the browser.
When you compare it to other libraries, you'll be able to tell that it performs better, and you can utilize Webpack with it, of course. Additionally, this library uses an imperative approach, which makes it difficult to work with complex layouts.
import { PDFDocument } from 'pdf-lib'
// PDF Create
const pdfDoc = await PDFDocument.create()
const page = pdfDoc.addPage()
page.drawText('Hello World')
const pdfBytes = await pdfDoc.save()
IronPDF
IronPDF for Node.js renders PDFs from HTML strings, files, and web URLs by using the robust Chrome Engine. It is advised to assign this operation to the server side since rendering can be computationally demanding. In order to offload the computational effort and await the outcome, frontend frameworks like ReactJs and Angular can communicate with the server. The outcome can then be shown on the front end side.
Software engineers may produce, modify PDF documents, and extract PDF material with the use of the IronPDF library, which was created and maintained by Iron Software.
When it comes to
the creation of PDF documents using HTML, URL, JavaScript, CSS, and a variety of image formats
A signature and headers should be included.
Add, Copy, Split, Merge, and Delete PDF Pages
Can able to include CSS properties.
Performance improvement Async and complete multithreading support
import {PdfDocument} from "@ironsoftware/ironpdf";
(async () => {
const pdf = await PdfDocument.fromHtml("<h1>Hello World</h1>");
await pdf.saveAs("Output.pdf");
})();