
August 16, 2023 08:08 by
Peter
In this post, we will look at how to use the @Input() decorator in Angular to share data from a parent component to a child component.
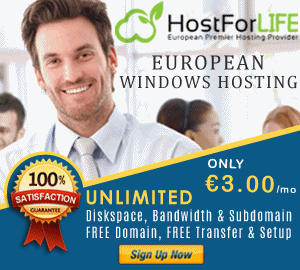
Recognizing the @Input() Decorator
The @Input() decorator allows you to transmit data from a parent component to a child component in Angular. It effectively establishes an input property on the child component that may be tied to a value in the template of the parent component. When the value of the input property in the parent component changes, the child component is automatically updated with the new value.
Configuring the Parent Component
Let's begin with a simple example. Consider the following scenario: we have a parent component that displays a user's name, and we wish to pass this name to a child component for display.
Using the Angular CLI, create a new parent component:
ng generate component parent
Open the parent.component.ts file and add the @Input() decorator to the following property:
import { Component } from '@angular/core';
@Component({
selector: 'app-parent',
template: `
<h1>Hello, {{ userName }}!</h1>
<app-child [inputName]="userName"></app-child>
`,
})
export class ParentComponent {
userName = 'Tahir Ansari';
}
Creating the Child Component
Now, let's create the child component that will receive and display the user's name.
Generate a child component using the Angular CLI:
ng generate component child
In the child.component.ts file, use the @Input() decorator to define an input property:
import { Component, Input } from '@angular/core';
@Component({
selector: 'app-child',
template: `
<p>Received name from parent: {{ receivedName }}</p>
`,
})
export class ChildComponent {
@Input() inputName: string;
get receivedName() {
return this.inputName;
}
}
Wiring Up the Module
ensure that you declare both the parent and child components in your module:
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { ParentComponent } from './parent.component';
import { ChildComponent } from './child.component';
@NgModule({
imports: [BrowserModule],
declarations: [ParentComponent, ChildComponent],
bootstrap: [ParentComponent],
})
export class AppModule {}
Conclusion
In this article, we've seen how to pass data from a parent component to a child component in Angular using the @Input() decorator. This feature allows for seamless communication between components and enables dynamic updates whenever the data in the parent component changes.
Angular's component-based architecture, combined with features like @Input(), empowers developers to build modular and maintainable applications by promoting the separation of concerns and reusability of components.