
June 5, 2023 09:46 by
Peter
You can now test our API endpoints using Visual Studio 17.5 with the aid of Visual Studio. Let's begin with an implementation example.
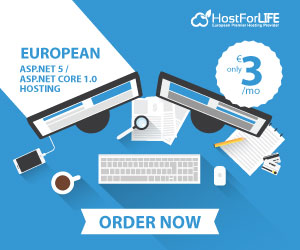
Step 1
Create a new Web API project using.NET Core.
Step 2
Create one API controller and multiple endpoints within the new project, which will be tested using an HTTP file.
using Microsoft.AspNetCore.Mvc;
using RudderStackDemo.Entities;
using RudderStackDemo.Repositories;
namespace RudderStackDemo.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class ProductsController : ControllerBase
{
private readonly IProductService _productService;
public ProductsController(IProductService productService)
{
_productService = productService;
}
/// <summary>
/// Product List
/// </summary>
/// <returns></returns>
[HttpGet]
public async Task<IActionResult> ProductListAsync()
{
var productList = await _productService.ProductListAsync();
if (productList != null)
{
return Ok(productList);
}
else
{
return NoContent();
}
}
/// <summary>
/// Get Product By Id
/// </summary>
/// <param name="productId"></param>
/// <returns></returns>
[HttpGet("{productId}")]
public async Task<IActionResult> GetProductDetailsByIdAsync(int productId)
{
var productDetails = await _productService.GetProductDetailByIdAsync(productId);
if (productDetails != null)
{
return Ok(productDetails);
}
else
{
return NotFound();
}
}
/// <summary>
/// Add a new product
/// </summary>
/// <param name="productDetails"></param>
/// <returns></returns>
[HttpPost]
public async Task<IActionResult> AddProductAsync(ProductDetails productDetails)
{
var isProductInserted = await _productService.AddProductAsync(productDetails);
if (isProductInserted)
{
return Ok(isProductInserted);
}
else
{
return BadRequest();
}
}
/// <summary>
/// Update product details
/// </summary>
/// <param name="productDetails"></param>
/// <returns></returns>
[HttpPut]
public async Task<IActionResult> UpdateProductAsync(ProductDetails productDetails)
{
var isProductUpdated = await _productService.UpdateProductAsync(productDetails);
if (isProductUpdated)
{
return Ok(isProductUpdated);
}
else
{
return BadRequest();
}
}
/// <summary>
/// Delete product by id
/// </summary>
/// <param name="productId"></param>
/// <returns></returns>
[HttpDelete]
public async Task<IActionResult> DeleteProductAsync(int productId)
{
var isProductDeleted = await _productService.DeleteProductAsync(productId);
if (isProductDeleted)
{
return Ok(isProductDeleted);
}
else
{
return BadRequest();
}
}
}
}
Step 3
Right-click on the project name in the solution and add the API-Test.http file inside your project.
Step 4
Add different HTTP endpoint requests inside the HTTP file.
GET https://localhost:7178/api/Products
###
@productId = 2
GET https://localhost:7178/api/Products/{{productId}}
###
POST https://localhost:7178/api/Products
Content-Type application/json
{
"id": 3,
"productName": "Laptop",
"productDescription": "HP Pavilion",
"productPrice": 80000,
"productStock": 10
}
###
PUT https://localhost:7178/api/Products
Content-Type application/json
{
"id": 3,
"productName": "Laptop",
"productDescription": "DELL",
"productPrice": 80000,
"productStock": 10
}
###
DELETE https://localhost:7178/api/Products?productId=1
Step 5
Now you can execute the individual API endpoint after clicking on the run button corresponding to each request as shown below.
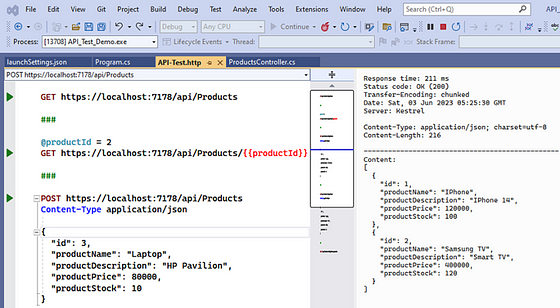