The concat operator is used in RxJS (Reactive Extensions for JavaScript) to concatenate multiple observables together, sequentially, in the order that they are supplied. It makes ensuring that emissions from observables are processed sequentially and that they are subscribed to.
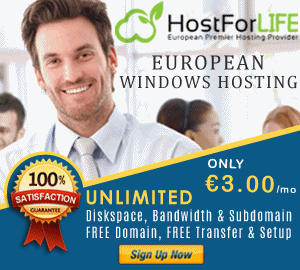
Set up RxJS
You can use npm to install RxJS if you haven't previously.
npm install rxjs
Import Concat and Any Other Essential Programs
To create observables, import the concat operator along with any additional functions or operators that you require. One way to build observables with values would be to utilize of.
import { concat, of } from 'rxjs';
Basic Syntax of RxJS
import { concat } from 'rxjs';
const resultObservable = concat(observable1, observable2, observable3, ...);
The concat function takes multiple observables as arguments, and it returns a new observable (resultObservable) that represents the concatenation of these observables.
Sequence of Action
- In the order that they are passed to concat, observables are subscribed to.
- Each observable's values are processed one after the other.
Awaiting Finalization
- Concat waits for every observable to finish before proceeding to the following one.
- Subsequent observables won't be subscribed to if an observable never completes, meaning it keeps emitting values forever.
Example
import { concat, of } from 'rxjs';
const observable1 = of(1, 2, 3);
const observable2 = of('A', 'B', 'C');
const resultObservable = concat(observable1, observable2);
resultObservable.subscribe(value => console.log(value));
Output
1 2 3 A B C
In this example, observable1 emits values 1, 2, and 3, and then observable2 emits values 'A', 'B', and 'C'. The concat operator ensures that the values are emitted in the specified order.
Use Cases
concat is useful when you want to ensure a sequential execution of observables.
It's commonly used when dealing with observables that represent asynchronous operations, and you want to perform these operations one after the other.
Error Handling
If any observable in the sequence throws an error, concat will stop processing the sequence, and the error will be propagated to the subscriber.
The concat operator in RxJS is a powerful tool for managing the sequential execution of observables, providing a clear order of operations and waiting for each observable to complete before moving on to the next one.