
February 1, 2024 08:13 by
Peter
The robust front-end framework Angular offers developers a number of tools to create dynamic and manageable user interfaces. Content projection is one such feature that greatly enhances UI flexibility and reusability. Through the dynamic injection of content into predetermined slots inside a component's template, content projection enables developers to design components that are both adaptable and reusable.
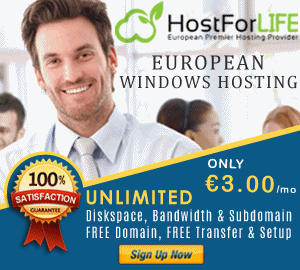
Comprehending Content Projection
A feature in Angular called content projection, or transclusion, allows content from a parent component to be inserted into a specific area of a child component. This method facilitates the creation of adaptable and adjustable user interfaces and encourages component reuse.
Content Projection's Advantages
Component Reusability: Content projection enables programmers to design components that may be applied to various areas of an application at different times. Content can be projected into designated slots to increase component versatility and adaptability to a range of use scenarios.
Simplified API: Rather than requiring intricate setups, components may expose a simplified API that accepts content as input. This improves code maintainability by making the components simpler for developers to use and comprehend.
Consistent Style and Layout: Content projection aids in preserving uniform styling and layout throughout an application's many sections. Components with specified slots can be designed by developers to guarantee that the content projection follows a consistent format.
Improved Concern Separation: Content projection promotes a distinct division of responsibilities between the parent and child components. Child components manage the content display, allowing parents to concentrate on business logic, thus fostering a modular and maintainable codebase.
Practical Use Case
Think of a basic card component that can show text, photos, or even bespoke components, among other kinds of information. We may construct a reusable card component that can adjust to various content kinds by using content projection.
card.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-card',
standalone: true,
imports: [],
template: `
<div class="card">
<div class="card-header">
<ng-content select="[card-header]"></ng-content>
</div>
<div class="card-body">
<ng-content select="[card-body]"></ng-content>
</div>
<div class="card-footer">
<ng-content select="[card-footer]"></ng-content>
</div>
</div>
`,
styles: [
'.card { border: 1px solid #ddd; border-radius: 8px; overflow: hidden; margin: 16px; }',
'.card-header, .card-footer { background-color: #f0f0f0; padding: 8px; }',
'.card-body { padding: 16px; }',
],
})
export class CardComponent {}
In this example, the CardComponent defines three slots: card-header, card-body, and card-footer. The content projected into these slots will be rendered within the corresponding sections of the card.
app.component.ts
import { CommonModule } from '@angular/common';
import { Component } from '@angular/core';
import { CardComponent } from './card/card.component';
@Component({
selector: 'app-root',
standalone: true,
imports: [CommonModule, CardComponent],
template: `
<app-card>
<div card-header>
<h2>Angular is awesome</h2>
</div>
<div card-body>
<p>Lets have a fun with it</p>
</div>
<div card-footer>
<button>Read More</button>
</div>
</app-card>
<app-card>
<div card-header>
<h2>Product Information</h2>
</div>
<div card-body>
<img
src="https://cdn-images-1.medium.com/v2/resize:fit:184/1*[email protected]"
alt="Product Image"
style="max-width: 100%"
/>
<ul>
<li>Feature 1</li>
<li>Feature 2</li>
<li>Feature 3</li>
</ul>
</div>
<div card-footer>
<button>Add to Cart</button>
</div>
</app-card>
`,
})
export class AppComponent {}