Minification and tree shaking are two fundamental techniques used in Angular (and other web development frameworks) to streamline and optimize code for best performance. These methods assist in decreasing the size of your application, which is essential for improved user experience and quicker load times. Now let's examine each of these procedures in relation to Angular:
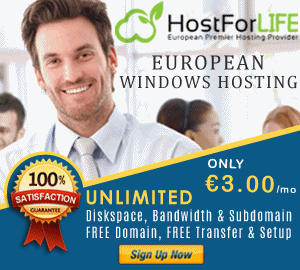
1. First off, what is minification?
Minification is the process of renaming variables to shorter names and eliminating characters from your code that aren't needed, including whitespace and comments. Your code files will download more quickly as a result of this smaller size. Typically, TypeScript is used to write Angular applications, and it is subsequently transpiled to JavaScript. The JavaScript code that results is subjected to the minification process.
This is how to make Angular's minification enabled.
A. Manufacturing Construct
To build your production application, you can use the build command provided by Angular CLI. The Angular CLI automatically applies minification when you build for production.
ng build --prod
This command generates a production-ready bundle with minified and optimized code.
B. The Terser Plugin
The Terser plugin, used for minification in Angular, provides with different configuration options. By including options in your angular.json file, you can alter the minification procedure.
"architect": {
"build": {
"options": {
"optimization": true,
"outputPath": "dist/my-app",
"terserOptions": {
"compress": {
"pure_funcs": ["console.log"],
"drop_console": true
},
"mangle": true
}
}
}
}
The functions that are pure and can be safely removed are specified using the pure_funcs parameter in the example above. All console statements are eliminated using the drop_console option, and variable names are obscured by setting mangle to true.
C. Compiling Angular AOT (Ahead-of-Time)
There are two compilation modes for Angular applications: Just-In-Time (JIT) and AOT (Ahead-of-Time). For production builds, AOT compilation is recommended since it enables greater tree shaking and optimization. Smaller bundle sizes are the outcome of its compilation of Angular templates and components during the construction process.
In your tsconfig.json file, you can set AOT compilation to run automatically.
"angularCompilerOptions": {
"fullTemplateTypeCheck": true,
"strictInjectionParameters": true
}
2. What Is Shaking of Trees?
The practice of removing unused or dead code from your program is called tree shaking. It contributes to reducing the bundle size by removing any unnecessary modules or code.
A. Configuration of the Module
Make sure the arrangement of your Angular modules permits efficient tree shaking. Dependencies and boundaries between modules should be obvious. To make the process of removing useless code easier, stay away from needless inter-module dependencies.
B. Analysis of Static Data
Tree shaking is most effective when combined with static analysis, so stay away from runtime code or dynamic imports that make it difficult for the build tool to identify which portions of the code are being utilized.
C. Mode of Production
Like minification, production builds yield the best results from tree shaking. Tree shaking is enabled automatically by Angular CLI when you execute the production build with the --prod flag.
ng build --prod
2. What Is Shaking of Trees?
The practice of removing unused or dead code from your program is called tree shaking. It contributes to reducing the bundle size by removing any unnecessary modules or code.
A. Configuration of the Module
Make sure the arrangement of your Angular modules permits efficient tree shaking. Dependencies and boundaries between modules should be obvious. To make the process of removing useless code easier, stay away from needless inter-module dependencies.
B. Analysis of Static Data
Tree shaking is most effective when combined with static analysis, so stay away from runtime code or dynamic imports that make it difficult for the build tool to identify which portions of the code are being utilized.
C. Mode of Production
Like minification, production builds yield the best results from tree shaking. Tree shaking is enabled automatically by Angular CLI when you execute the production build with the --prod flag.
ng build --prod
D. Angular Dependency Injection
The dependency injection system in Angular occasionally causes issues with tree shaking. Steer clear of injecting components or services that aren't needed in a certain module. This guarantees that the services that are not utilized are excluded from the final package.
E. Renderer of Angular Ivy
Angular Ivy, the new rendering engine introduced in Angular 9, adds enhancements to tree shaking. Better static analysis capabilities enable more efficient removal of dead code during the build process.
Configuring Webpack (for Advanced Users):
You can alter the webpack configuration that the Angular CLI uses if you require further control over the build process. This creates a webpack.config.js file in your project and involves ejecting the webpack configuration. Utilize this file to adjust code splitting and optimization, among other build process components.
In order to remove the webpack setup:
ng eject
Remember that ejecting is final and that you will be in charge of keeping the webpack settings updated.
In summary
In summary, minification and tree shaking are combined to optimize Angular apps. Achieving optimal performance requires configuring Terser's settings, turning on AOT compilation, properly structuring modules, and being aware of the limitations of tree shaking. Make sure the optimizations don't affect your application's functioning by conducting extensive testing.