
January 10, 2024 07:07 by
Peter
Setting environment variables in an AngularJS application happens at the configuration stage; this usually takes place in a separate file containing the configuration data. This is an illustration of how to set environment variables in an AngularJS application:
First Step
To save the environment variables, create a file in the root directory of your application, say config.js.
Step Two
Use the following code to define the environment variables in the file:
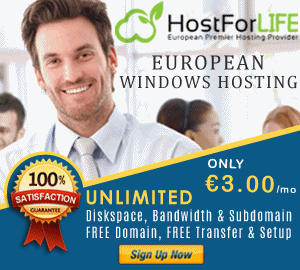
"use strict";
angular.module('config', [])
.constant('ENV', {name:'development',tokenURL:'http://localhost:62511/token',apiURL:'http://localhost:62511/api',biUrl:'http://localhost:4200/',backgroundimage:'../images/backgroundimage.jpg',logo:'images/ogo.png'});
Step 3
Include the config.js file in your HTML file, just like any other JavaScript file:
<script src="scripts/index.config.js"></script>
Step 4
Inject the env constant in your AngularJS controllers, services, or directives to access the environment variables,
angular.module("myApp").controller("MyController", function($scope, env) {
console.log(env.apiUrl);
console.log(env.debugEnabled);
});
development: {
options: {
dest: '<%= yeoman.app %>/scripts/config.js'
},
constants: {
ENV: {
name: 'development',
tokenURL: "http://localhost:62511/token",
apiURL: "http://localhost:62511/api",
biUrl: "http://localhost:4200/",
backgroundimage: "../images/backgroundimage.jpg",
logo: "images/logo.png",
}
}
},
qa: {
options: {
dest: '<%= yeoman.dist %>/scripts/config.js'
},
constants: {
ENV: {
name: 'qa',
tokenURL: "https://qa.hostforlife.eu/token",
apiURL: "https://qa.hostforlife.eu/api",
biUrl: "https://qa-dashboard.hostforlife.eut/",
backgroundimage: "../images/backgroundimage.jpg",
logo: "images/logo.png",
}
}
},
Grunt command to run the Application,
grunt build --qa