
July 1, 2024 08:46 by
Peter
With the help of Angular's robust data binding capability, developers can synchronize data between the model and the view. Data binding is one of the ways that Angular enables communication between components and the DOM.
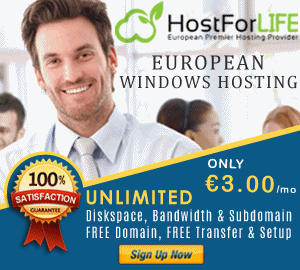
An outline of the primary Angular data binding types can be found here.
1. The use of interpolation
You can insert expressions between the double curly braces ({{ }}) by using interpolation. Data from the component is usually displayed to the view via this.
Usage: {{ expression }}
Example
<button (click)="onClick()">Click me</button>
2. Property Binding
Property binding allows you to bind data from the component to the property of an HTML element or directive. This helps in setting element properties based on component data.
Usage: [property]="expression"
Example
<img [src]="imageUrl">
3. Event Binding
Event binding allows you to respond to user events such as clicks, key presses, and mouse movements. It binds a DOM event to a method in the component.
Usage: (event)="handler"
Example
<button (click)="onClick()">Click me</button>
4. Two-Way Data Binding
Two-way data binding combines property binding and event binding to synchronize data between the model and the view. Angular provides the ngModel directive to facilitate two-way data binding.
Usage: [(ngModel)]="property"
Example
<input [(ngModel)]="name">
<p>Hello, {{ name }}!</p>
5. Detailed Explanation and Examples
Interpolation: Interpolation evaluates an expression in the context of the current data-bound component. It is useful for embedding dynamic values into the HTML.
Example
export class AppComponent {
title = 'Hello, Angular!';
}
<h1>{{ title }}</h1>
6. Property Binding
Property binding is used to set a property of a view element. It is a one-way data-binding from the component to the view.
Example
export class AppComponent {
imageUrl = 'https://angular.io/assets/images/logos/angular/angular.svg';
}
<img [src]="imageUrl">
7. Event Binding
Event binding listens to events such as keystrokes, mouse movements, clicks, and touches. When the event is triggered, it calls the specified method in the component.
Example
export class AppComponent {
onClick() {
console.log('Button clicked!');
}
}
<button (click)="onClick()">Click me</button>
8. Two-Way Data Binding
Two-way data binding allows for the automatic synchronization of data between the model and the view. The ngModel directive simplifies this by updating the model whenever the input value changes and updating the input value when the model changes.
Example
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: `
<input [(ngModel)]="name">
<p>Hello, {{ name }}!</p>
`
})
export class AppComponent {
name: string = '';
}
Conclusion
Data binding in Angular facilitates seamless communication between the template (view) and the component (model). It simplifies the process of displaying data, responding to user input, and keeping the view and model synchronized. For more detailed information, you can refer to the official Angular documentation on data binding: Angular Data Binding.