
June 13, 2024 09:09 by
Peter
Embedding external material within your Angular component is the process of working with iframes in Angular. You can use the HTML element <iframe> to accomplish this. If necessary, you can also manipulate and engage with the iframe directly from your Angular component. The procedures for integrating and using iframes in an Angular application are listed below.
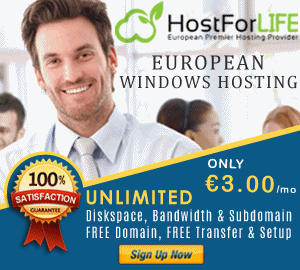
1. Fundamental Configuration
If you don't currently have an Angular component, start by creating one.
ng generate component iframe-example
2. Embed an Iframe
You can embed an iframe in the component template. Here is a simple example to embed an external website:
iframe-example.component.html
<div class="iframe-container">
<iframe [src]="iframeUrl" width="100%" height="600px"></iframe>
</div>
iframe-example.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-iframe-example',
templateUrl: './iframe-example.component.html',
styleUrls: ['./iframe-example.component.css']
})
export class IframeExampleComponent {
iframeUrl: string = 'https://www.example.com';
}
3. Dynamic Iframe URLs
You might want to load different URLs dynamically. You can bind the src attribute to a property in your component:
iframe-example.component.html
<div class="iframe-container">
<iframe [src]="iframeUrl" width="100%" height="600px"></iframe>
</div>
<button (click)="loadUrl('https://www.example.com')">Load Example</button>
<button (click)="loadUrl('https://www.angular.io')">Load Angular</button>
iframe-example.component.ts
import { Component, Sanitizer, SecurityContext } from '@angular/core';
import { DomSanitizer } from '@angular/platform-browser';
@Component({
selector: 'app-iframe-example',
templateUrl: './iframe-example.component.html',
styleUrls: ['./iframe-example.component.css']
})
export class IframeExampleComponent {
iframeUrl: any;
constructor(private sanitizer: DomSanitizer) {
this.iframeUrl = this.sanitizer.bypassSecurityTrustResourceUrl('https://www.example.com');
}
loadUrl(url: string) {
this.iframeUrl = this.sanitizer.bypassSecurityTrustResourceUrl(url);
}
}
4. Styling the Iframe
You can add styles to the iframe to ensure it fits well within your layout:
iframe-example.component.css
.iframe-container {
position: relative;
width: 100%;
height: 600px;
}
iframe {
border: none;
width: 100%;
height: 100%;
}
5. Interacting with the Iframe
Interacting with the iframe content can be more complex, especially when dealing with different domains due to security constraints (same-origin policy). Here’s an example of how you can post a message to the iframe:
iframe-example.component.html
<div class="iframe-container">
<iframe #myIframe [src]="iframeUrl" width="100%" height="600px"></iframe>
</div>
<button (click)="sendMessage()">Send Message</button>
iframe-example.component.ts
import { Component, ViewChild, ElementRef } from '@angular/core';
import { DomSanitizer } from '@angular/platform-browser';
@Component({
selector: 'app-iframe-example',
templateUrl: './iframe-example.component.html',
styleUrls: ['./iframe-example.component.css']
})
export class IframeExampleComponent {
@ViewChild('myIframe', { static: false }) myIframe: ElementRef;
iframeUrl: any;
constructor(private sanitizer: DomSanitizer) {
this.iframeUrl = this.sanitizer.bypassSecurityTrustResourceUrl('https://www.example.com');
}
sendMessage() {
const iframeWindow = this.myIframe.nativeElement.contentWindow;
iframeWindow.postMessage('Hello from Angular', '*');
}
}
In the iframe content (if you control it), you can listen for messages:
Content inside the iframe (example.com).
window.addEventListener('message', (event) => {
console.log('Message received from parent:', event.data);
});
6. Handling messages from the Iframe
You can also listen to messages sent from the iframe to your Angular application:
iframe-example.component.ts
import { Component, HostListener } from '@angular/core';
import { DomSanitizer } from '@angular/platform-browser';
@Component({
selector: 'app-iframe-example',
templateUrl: './iframe-example.component.html',
styleUrls: ['./iframe-example.component.css']
})
export class IframeExampleComponent {
iframeUrl: any;
constructor(private sanitizer: DomSanitizer) {
this.iframeUrl = this.sanitizer.bypassSecurityTrustResourceUrl('https://www.example.com');
}
@HostListener('window:message', ['$event'])
onMessage(event: MessageEvent) {
if (event.origin !== 'https://www.example.com') {
return;
}
console.log('Message received from iframe:', event.data);
}
}
Conclusion
By following these steps, you can effectively embed and interact with iframes in your Angular application. Remember to handle security concerns, such as sanitizing URLs and ensuring that message passing complies with the same-origin policy. This approach allows you to integrate external content and communicate with iframes in a seamless manner.