
August 14, 2024 07:19 by
Peter
In Angular, component routing is usually done via the Router service. This allows you to call or go to a component from another component. Here's how you can make this happen. In the Router Module, define routes. The first step is to configure your routes in your AppRoutingModule (or any other defined routing module). Describe the pathways and the matching parts.
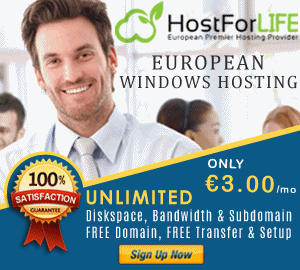
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
import { ComponentA } from './component-a/component-a.component';
import { ComponentB } from './component-b/component-b.component';
const routes: Routes = [
{ path: 'component-a', component: ComponentA },
{ path: 'component-b', component: ComponentB },
// Add more routes as needed
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
Use RouterLink in Templates
You can navigate to another component using the RouterLink directive in your template (HTML) of the first component.
<!-- component-a.component.html -->
<a [routerLink]="['/component-b']">Go to Component B</a>
Navigate Programmatically Using the Router
You can also navigate programmatically using the Angular Router in your component's TypeScript file.
import { Component } from '@angular/core';
import { Router } from '@angular/router';
@Component({
selector: 'app-component-a',
templateUrl: './component-a.component.html',
styleUrls: ['./component-a.component.css']
})
export class ComponentA {
constructor(private router: Router) { }
goToComponentB() {
this.router.navigate(['/component-b']);
}
}
In HTML template
<!-- component-a.component.html -->
<button (click)="goToComponentB()">Go to Component B</button>
Outlet to Render Routed Components
Ensure you have a <router-outlet></router-outlet> in your main HTML template (e.g., app.component.html) where the routed components will be displayed.
<!-- app.component.html -->
<router-outlet></router-outlet>
Lazy Loading (Optional)
If you want to load a module lazily, define your route like this.
const routes: Routes = [
{
path: 'component-b',
loadChildren: () =>
import('./component-b/component-b.module')
.then(m => m.ComponentBModule)
}
];
Summary
- RouterLink: For navigation within the template.
- Router.navigate: For programmatic navigation within the component's TypeScript file.
- Router Outlet: Acts as a placeholder in the template where routed components are displayed.
This setup allows you to call or navigate between components effectively in Angular.