
August 22, 2023 12:06 by
Peter
The @Output decorator is used in this article to share data from child to parent components in Angular. We'll go through the fundamental ideas underlying this technique, show how it works in practice using examples, and explain its benefits in terms of code organization, reusability, and maintainability. By the end of this article, you'll have a solid grasp of how to use the @Output decorator to add efficient data flow methods to your Angular apps.
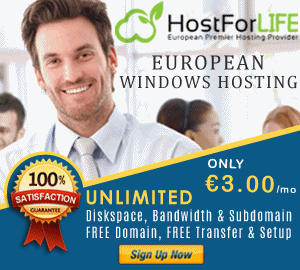
Understanding Interaction of Components
Components are the building blocks of the user interface in Angular apps. They can be organized hierarchically, with parent components encapsulating child components.
These components frequently need to communicate with one another, transmitting data back and forth. While data sharing between parent and child components is very simple using input attributes, data sharing between child and parent components requires a different technique.
The @Output Decorator is a feature of Angular that allows communication between child and parent components to be more efficient. To emit custom events from the child component, it is used in conjunction with Angular's EventEmitter class. The parent component is aware of these events and can respond appropriately.
Step-by-Step Instructions
Let's go over how to use @Output in Angular to implement data sharing from child to parent components step by step.
Step 1: Make a Child Component
Create the child component first.
ng generate component child
In the child component HTML file (child.component.html), include a button to trigger the data emission.
<button (click)="sendData()">Send Data</button>
In the child component TypeScript file (child.component.ts), import the necessary modules, and create an @Output property and an instance of EventEmitter to emit the data.
import { Component, EventEmitter, Output } from '@angular/core';
@Component({
selector: 'app-child',
templateUrl: './child.component.html'
})
export class ChildComponent {
@Output() dataEvent = new EventEmitter<string>();
sendData() {
this.dataEvent.emit('Hello from child!');
}
}
Step 2. Use the Child Component in the Parent
Now, use the child component in the parent component HTML file (parent.component.html).
<app-child (dataEvent)="receiveData($event)"></app-child>
<div>{{ receivedData }}</div>
In the parent component TypeScript file (parent.component.ts), define the method to handle the emitted event.
import { Component } from '@angular/core';
@Component({
selector: 'app-parent',
templateUrl: './parent.component.html'
})
export class ParentComponent {
receivedData: string = '';
receiveData(data: string) {
this.receivedData = data;
}
}
In conjunction with EventEmitter, Angular's @Output decorator provides a strong mechanism for passing data from child to parent components. You can enable effective communication and interaction between different portions of your program by broadcasting custom events from child components. By fostering a clear separation of concerns, this strategy improves the modularity and maintainability of your system.
This pattern remains a core strategy for component interaction in Angular. Developers may create more dynamic and responsive user interfaces in their Angular applications by mastering the art of exchanging data with @Output.