I'm a slack backend.net developer. I have some knowledge of.net technologies, however I'm not very knowledgeable with Angular, a frontend JavaScript framework. In order to enable external communication between the front-end and RESTful web API services, my manager instructed me to jot down certain typescript codes. You all know what would happen if I told him I didn't know how to do it. Anyhow, let's return to the primary topic. Please be aware that this is only intended for novice users who want to fire once and forget. Please don't hold me responsible. My knowledge of typescripts is rather limited.
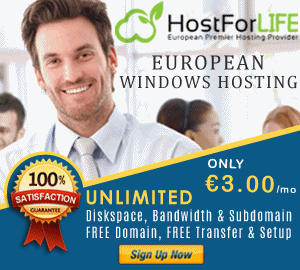
Resolution and Schematic Design
- I divided the entire assignment into four sections at first:
- Examining the options in the app.
- Setting up an environment depends on whether it's for production, QA, or development.
- Establishing a connection with external RESTful web API services.
- Requests to the services that include instructions or questions.
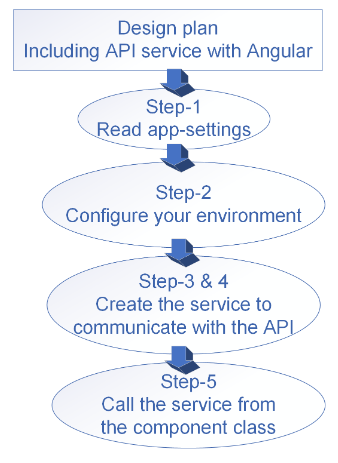
Basic knowledge - I guess you know it. So, in short.
- Restful web API: it’s an interface for communication with two or more applications.
- TypeScript: Compared to javaScript, It supports writing code with object-oriented concepts.
- Singleton design pattern: It creates a single shared instance of the object.
- Dependency injection design pattern: It is a style of object configuration in which objects are set by an external entity.
Explanation of each of the steps
- Step 1: Read all the configuration at runtime from the appSettings.json file so that applications can use this data at runtime.
- Step 2: Check the environment. Is it development, QA, or production? Set the environment settings data that you got from step 1.
- Step 3: Create a base class for the service to get the API service, for instance. Design it using the concept of the singleton design pattern. Get the settings data from step 2.
- Step 4: The main service class calls all the get/post/put/patch/delete required requests to the RESTful web API. Add the base class from step 3.
- Step 5: Use the dependency Injection pattern to inject the dependent service. Inject the dependent service class into the component class. Call the required methods of the service from the component class. Use the class from step 4.
Implementation of each of the classes using typeScript in the Angular project
- appsettings-json-reader package: Read all the data from the JSON file.
- npm install appsettings-json-reader
Axios package: Promise-based API method called.
npm install axios --save
I guess you already know how to implement step1 & step2.
Implementing steps 3
Creating the base class using a command.
ng g class BaseApiClientService
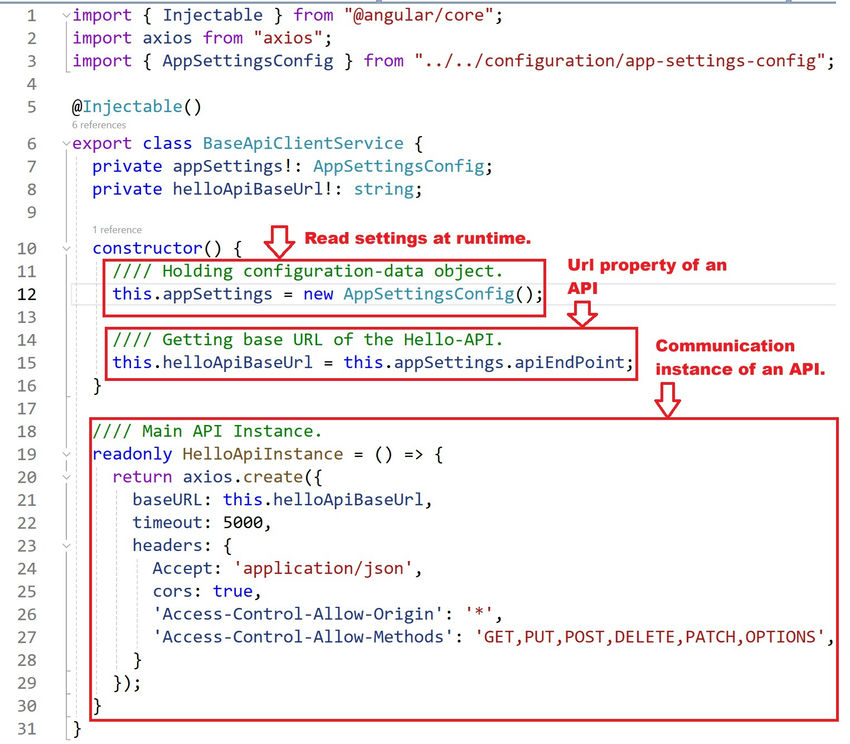
import { Injectable } from "@angular/core";
import axios from "axios";
import { AppSettingsConfig } from "../../configuration/app-settings-config";
@Injectable()
export class BaseApiClientService {
private appSettings!: AppSettingsConfig;
private helloApiBaseUrl!: string;
constructor() {
// Holding configuration-data object.
this.appSettings = new AppSettingsConfig();
// Getting base URL of the Hello-API.
this.helloApiBaseUrl = this.appSettings.apiEndPoint;
}
// Main API Instance.
readonly HelloApiInstance = () => {
return axios.create({
baseURL: this.helloApiBaseUrl,
timeout: 5000,
headers: {
Accept: 'application/json',
cors: true,
'Access-Control-Allow-Origin': '*',
'Access-Control-Allow-Methods': 'GET, PUT, POST, DELETE, PATCH, OPTIONS',
}
});
}
}
Implementing steps 4
Creating the service class using a command.
ng g service Hello-Api-Service
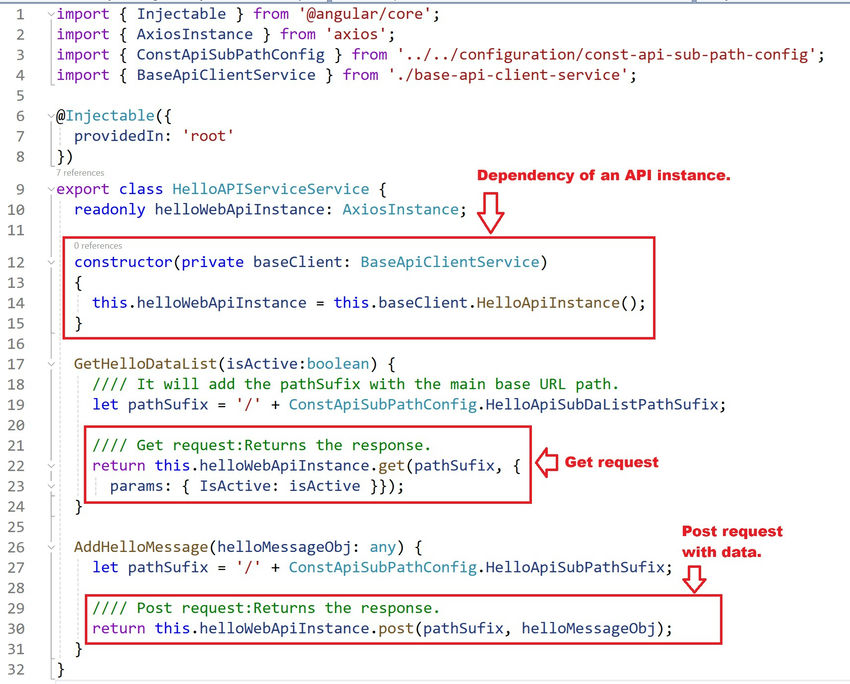
import { Injectable } from '@angular/core';
import { AxiosInstance } from 'axios';
import { ConstApiSubPathConfig } from '../../configuration/const-api-sub-path-config';
import { BaseApiClientService } from './base-api-client-service';
@Injectable({
providedIn: 'root'
})
export class HelloAPIServiceService {
readonly helloWebApiInstance: AxiosInstance;
constructor(private baseClient: BaseApiClientService)
{
this.helloWebApiInstance = this.baseClient.HelloApiInstance();
}
GetHelloDataList(isActive:boolean) {
//// It will add the pathSufix with the main base URL path.
let pathSufix = '/' + ConstApiSubPathConfig.HelloApiSubDaListPathSufix;
//// Get request:Returns the response.
return this.helloWebApiInstance.get(pathSufix, {
params: { IsActive: isActive }});
}
AddHelloMessage(helloMessageObj: any) {
let pathSufix = '/' + ConstApiSubPathConfig.HelloApiSubPathSufix;
//// Post request:Returns the response.
return this.helloWebApiInstance.post(pathSufix, helloMessageObj);
}
}
Implementing Steps 5
Creating the component class using a command.
ng g component HelloServiceGrid --module app
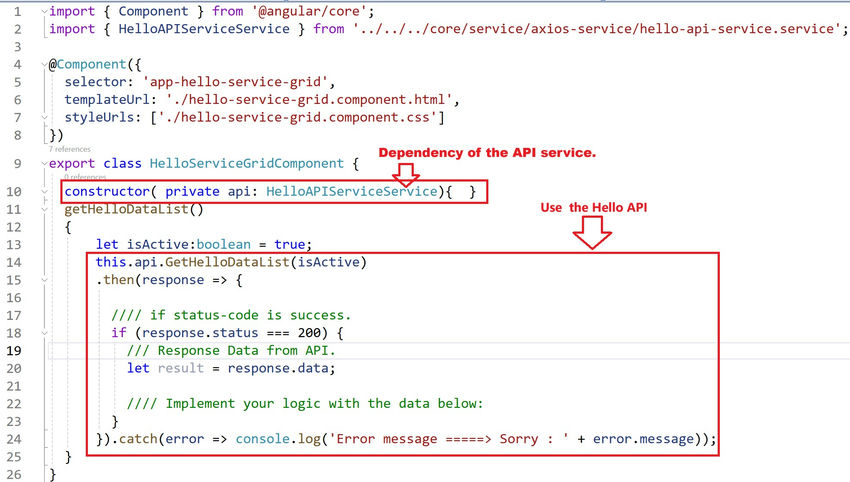
import { Component } from '@angular/core';
import { HelloAPIServiceService } from '../../../core/service/axios-service/hello-api-service.service';
@Component({
selector: 'app-hello-service-grid',
templateUrl: './hello-service-grid.component.html',
styleUrls: ['./hello-service-grid.component.css']
})
export class HelloServiceGridComponent {
constructor( private api: HelloAPIServiceService){ }
getHelloDataList()
{
let isActive:boolean = true;
this.api.GetHelloDataList(isActive)
.then(response => {
//// if status-code is success.
if (response.status === 200) {
/// Response Data from API.
let result = response.data;
//// Implement your logic with the data below:
}
}).catch(error => console.log('Error message =====> Sorry : ' + error.message));
}
}
Don’t forget to check the App.module.ts file. Your components should be added to the declarations array, and your services should be added to the providers array.
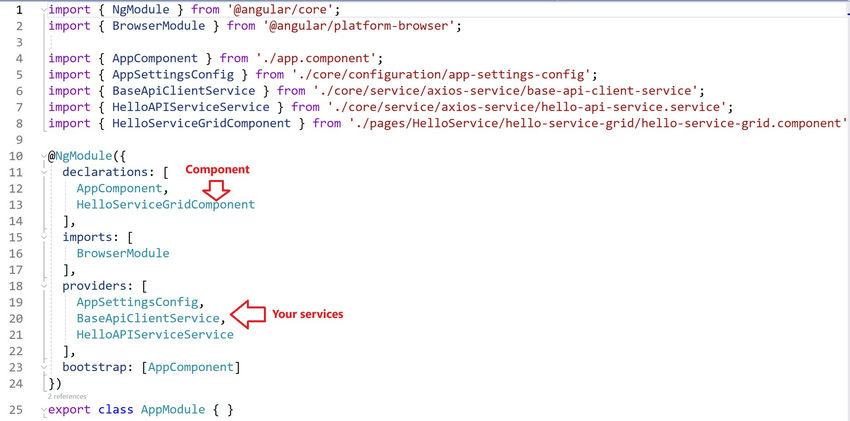
I'll go over how to define environmental configurations and read configurations at runtime in the next section, which covers leveraging Azure DevOps CI/CD pipelines to deploy an Angular app to the Azure environment. Anyhow, I have to leave.