You will discover the solutions to the following questions and how to transfer/share data from the child component to the parent component in this walkthrough.
- @Output: What is it?
- An Event Emitter: What Is It?
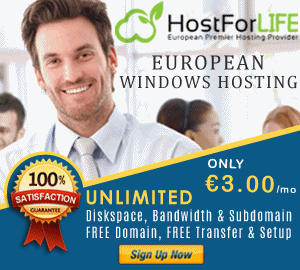
What is @Output?
@Output decorator is used to share the data from the child component to the parent component.
What is an event emitter?
An event emitter is used to create custom events for AngularComponent. Mainly, the event emitter is used to pass data from a child component to a parent component using the @output decorator.
In short, sending data to the parent is publishing /emitting the vent, and the parent component listens the same.
Send Data from Child to Parent Component
Create an Angular Project called “AnguWalk” using the following CLI command.
Command
ng new AnguWalk
Example
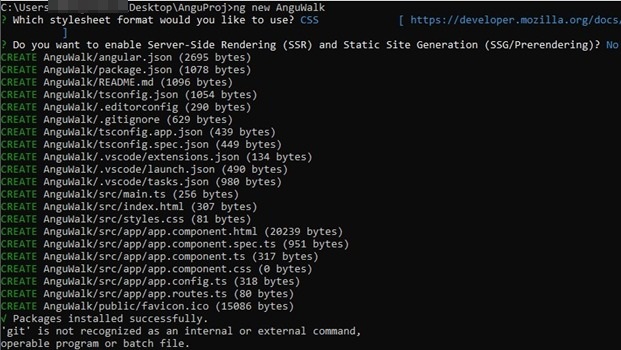
Move the cursor to inside the project folder and open Visual Studio code.
Command
cd anguwalk <enter>
code . <enter>
Example

Note. Visual studio code will get started only if your system is configured with path and settings.
Now, we are going to Create a child component to issue the default city value.
Command
ng g c childdefacity
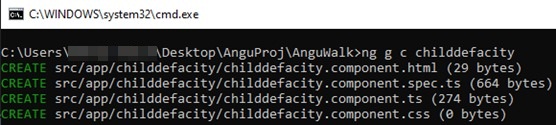
Open the childdefacity.component.ts file and update the following code.
import { Component, EventEmitter, OnInit, Output } from '@angular/core';
@Component({
selector: 'app-childdefacity',
standalone: true,
imports: [],
templateUrl: './childdefacity.component.html',
styleUrls: ['./childdefacity.component.css']
})
export class ChilddefacityComponent implements OnInit {
defaultcity: string = "Shirdi";
@Output() updatecity = new EventEmitter<string>();
ngOnInit(): void {}
SendToParent() {
this.updatecity.emit(this.defaultcity);
}
}
Open the childdefacity.component.html file and update the following code.
<p>Child Component Content --> childdefacity works!</p>
<button type="button" style="font-size: xx-large;" (click)="SendToParent()">Send Default City</button>
Open the app.component.ts file and update the following code.
import { Component, NgModule, OnInit } from '@angular/core';
import { FormsModule } from '@angular/forms';
import { CommonModule } from '@angular/common';
import { RouterOutlet } from '@angular/router';
import { ChilddefacityComponent } from './childdefacity/childdefacity.component';
declare function HelloMsg(arg: any): void;
@Component({
selector: 'app-root',
standalone: true,
imports: [RouterOutlet, FormsModule, CommonModule, ChilddefacityComponent],
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
parentdefacity: string = '';
txtFullname: string = '';
txtCityname: string = '';
getdata(defacity: string) {
this.txtCityname = defacity;
// alert(this.txtCityname);
}
formpro(form: any) {
alert('submit');
console.log(form);
console.log(this.txtCityname);
console.log(this.txtFullname);
}
}
Open the app.component.ts file and update the following code.
import { Component, NgModule, OnInit } from '@angular/core';
import { FormsModule } from '@angular/forms';
import { CommonModule } from '@angular/common';
import { RouterOutlet } from '@angular/router';
import { ChilddefacityComponent } from './childdefacity/childdefacity.component';
declare function HelloMsg(arg: any): void;
@Component({
selector: 'app-root',
standalone: true,
imports: [RouterOutlet, FormsModule, CommonModule, ChilddefacityComponent],
templateUrl: './app.component.html',
styleUrl: './app.component.css'
})
export class AppComponent {
parentdefacity: string = "";
txtFullname: string = "";
txtCityname: string = "";
getdata(defacity: string) {
this.txtCityname = defacity;
// alert(this.txtCityname);
}
formpro(form: any) {
alert("submit");
console.log(form);
console.log(this.txtCityname);
console.log(this.txtFullname);
}
}
Open the app.component.html file and update the following code.
<router-outlet />
<form #contForm="ngForm" (ngSubmit)="formpro(contForm.value)">
<table>
<tr>
<td>Fullname</td>
<td>
<input
type="text"
id="txtFullname"
style="font-size: xx-large;"
name="txtFullname"
[(ngModel)]="txtFullname">
</td>
</tr>
<tr>
<td>City</td>
<td>
<input
type="text"
id="txtCity"
style="font-size: xx-large;"
name="txtCity"
[(ngModel)]="txtCityname">
</td>
</tr>
<tr>
<td colspan="2">
<app-childdefacity (updatecity)="getdata($event)"></app-childdefacity>
</td>
</tr>
<tr>
<td colspan="2">
<button type="submit" style="font-size: xx-large;">Submit</button>
</td>
</tr>
</table>
</form>
OUTPUT
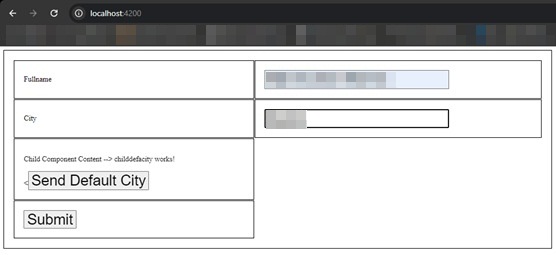