
May 15, 2024 08:44 by
Peter
The RxJS library's Angular module's startWith() operator is used to prepend a starting value or set of values to the start of an observable series. This operator makes sure that these initial values are released as soon as the observable is subscribed, before it starts to release its typical series of values.
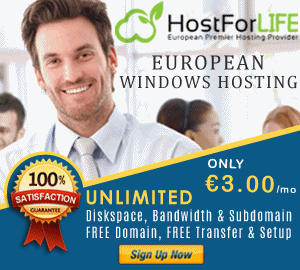
Setting up
Make sure your Angular project has RxJS installed. If it's not installed already, npm can be used to add it:
Usage
Import the startWith operator from RxJS in the component or service where you want to use it:
import { startWith } from 'rxjs/operators';
Example
Let's illustrate how startWith() works with a practical example.
Service Definition
Assume you have a service that provides an observable stream of numbers:
import { Injectable } from '@angular/core';
import { Observable, interval } from 'rxjs';
@Injectable({
providedIn: 'root'
})
export class NumberService {
getNumbers(): Observable<number> {
return interval(1000); // Emits incremental numbers every second
}
}
Component Implementation
In your component, you can consume this service and use the startWith() operator to prepend an initial value before the stream of numbers starts emitting.
import { Component, OnInit } from '@angular/core';
import { NumberService } from './number.service';
import { startWith } from 'rxjs/operators';
@Component({
selector: 'app-number-display',
template: `
<h2>Number Stream</h2>
<ul>
<li *ngFor="let number of numberStream$ | async">{{ number }}</li>
</ul>
`
})
export class NumberDisplayComponent implements OnInit {
numberStream$: Observable<number>;
constructor(private numberService: NumberService) { }
ngOnInit(): void {
this.numberStream$ = this.numberService.getNumbers().pipe(
startWith(0) // Prepend 0 as the initial value
);
}
}
In this example
- this.numberService.getNumbers() returns an observable that emits incremental numbers every second using interval(1000).
- startWith(0) is used within the pipe() method to prepend the initial value 0 to the beginning of the observable sequence. This means that 0 will be emitted immediately upon subscription, before the interval starts emitting numbers.
Template Usage
In the component's template (number-display.component.html), subscribe to numberStream$ using the async pipe to display the emitted numbers:
<h2>Number Stream</h2>
<ul>
<li *ngFor="let number of numberStream$ | async">{{ number }}</li>
</ul>