
July 16, 2024 07:51 by
Peter
Sanitization in Angular is an essential component of web development that guards against Cross-Site Scripting (XSS) assaults, ensuring the security of the application. Angular comes with built-in capabilities to sanitize data before it's rendered in the application, shielding it from malicious scripts and safeguarding users.
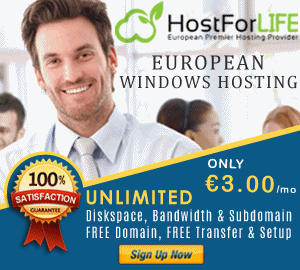
Recognizing XSS and the Sanitization Need
When a hacker inserts malicious scripts into content from an unreliable source, it's known as an XSS assault. These scripts have the ability to run in the user's browser and may steal information or carry out unauthorized tasks. Angular sanitizes inputs prior to binding them to the DOM in order to reduce these dangers. The integrated sanitization feature of Angular verifies that user data is free of dangerous information.
How Angular Handles Sanitization?
Angular sanitizes data automatically in templates for certain contexts.
- HTML: When binding data to HTML, Angular sanitizes it to prevent the injection of malicious HTML.
- Styles: Angular ensures that style bindings are safe.
- URLs: URL bindings are sanitized to avoid malicious links.
- Resource URLs: When loading resources, Angular ensures URLs are safe.
Using Angular's DomSanitizer
For cases where you need to allow specific trusted content, Angular provides the DomSanitizer service. This service allows you to mark content as safe explicitly.
Example. Using DomSanitizer
Let's create an example where we use DomSanitizer to handle potentially unsafe HTML content safely.
Step 1. Set Up the Angular Project
Create a new Angular project if you don't have one already.
Step 2. Create a Component
Generate a new component for our example.
Step 3. Implement Sanitization in the Component
Modify the safe-content.component.ts to use DomSanitizer.
import { Component } from '@angular/core';
import { DomSanitizer, SafeHtml } from '@angular/platform-browser';
@Component({
selector: 'app-safe-content',
templateUrl: './safe-content.component.html',
styleUrls: ['./safe-content.component.css']
})
export class SafeContentComponent {
public trustedHtml: SafeHtml;
constructor(private sanitizer: DomSanitizer) {
const potentiallyUnsafeHtml = `<div style="color: red;">This is safe content</div>`;
this.trustedHtml = this.sanitizer.bypassSecurityTrustHtml(potentiallyUnsafeHtml);
}
}
In this example, we use bypassSecurityTrustHtml to mark the HTML content as safe explicitly.
Step 4. Display the Content in the Template
Modify safe-content.component.html to display the sanitized HTML content.
<div [innerHtml]="trustedHtml"></div>
Step 5. Use the Component in Your App
Include the SafeContentComponent in your app.component.html
<app-safe-content></app-safe-content>
Step 6. Run the Application
Run your Angular application to see the sanitized content in action.
Conclusion
Web security requires sanitization, and Angular offers powerful tools for managing it. Through the use of DomSanitizer and Angular's automated sanitization, developers can guarantee that their apps are safe from cross-site scripting (XSS) threats while maintaining flexibility in content rendering. Building dependable and safe online apps requires an understanding of and adherence to Angular sanitization procedures.
You may handle user-generated content in your Angular applications safely by following the instructions in this article. This will guarantee that your application is secure and that the data of your users is safeguarded.