
May 25, 2023 13:02 by
Peter
To sort an array of objects by a particular date column in ascending or descending order using a custom pipe in Angular, you can modify the date-sort.pipe.ts file as follows:
Custom Pipe (date-sort.pipe.ts)
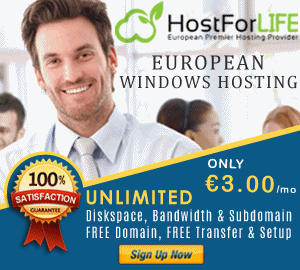
In Pipe declaration, we can define the name of the filter (pipe), When pipe is true, the pipe is pure, meaning that the transform() method is invoked only when its input arguments change. Pipes are pure by default.
In the transform() method, we can pass the array which needs to be filtered. Property parameter can as based on which date column we need to sort; ordering 'asc' | 'desc' can be also passed.
@Pipe({
name: 'orderByDate',
pure:false
})
export class OrderByDatePipe implements PipeTransform {
transform(array: any[], property: string, order: 'asc' | 'desc'): any[] {
if (!Array.isArray(array) || !property) {
return array;
}
array.sort((a, b) => {
const dateA = new Date(a[property]);
const dateB = new Date(b[property]);
if (order === 'asc') {
return dateA.getTime() - dateB.getTime();
} else {
return dateB.getTime() - dateA.getTime();
}
});
return array;
}
}
App.Module.ts for a particular module
Register the custom pipe in the module where you want to use it. For example, open the app.module.ts file and import and add OrderByDatePipe to the declarations array:
@NgModule({
declarations: [....,....],
imports: [
OrderByDatePipe
],
providers: [ ....]
For use across Application
1. We can use Share.Module.ts, Register the custom pipe in share module,
@NgModule({
declarations: [
OrderByDatePipe
],
imports: [
CommonModule
]
,exports:[
OrderByDatePipe
]
})
2. We can import ShareModule in whether we want to use it inside any module.
@NgModule({
declarations: [...,.... ],
imports: [
.....
SharedModule,
....
],
providers: [ .....]
App.Component.html
In your component's template, pass the desired date column and order ('asc' or 'desc') as parameters to the OrderByDatePipe :
Make sure to replace 'scheduleStartDate' with the actual name of the date column in your array of objects.
By providing the specific property/column to the orderByDate pipe, you can sort the array based on that property's date values in either ascending or descending order.
<div *ngFor="let schedule of CurrentSchedule | orderByDate:'scheduleStartDate':'asc'">
....
....
</div>
<div *ngFor="let schedule of CurrentSchedule | orderByDate:'scheduleStartDate':'desc'">
....
....
</div>