
June 8, 2023 09:58 by
Peter
To sort an array of objects by a checkbox selection in a column using a custom pipe in Angular, you can modify the checkbox-selection.pipe.ts file as follow
Custom Pipe (checkbox-selection.pipe.ts)
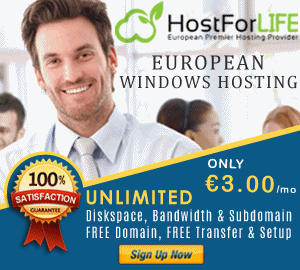
To generate pipe, use the following comment.
ng g p CheckboxSelectionPipe
g- stands for generate
p- stands for pipe
CheckboxSelectionPipe - use your custom pipe name instead of [checkboxslectionpipe]
In Pipe declaration, we can define the filter's name (pipe); when the pipe is true, the pipe is pure, meaning that the transform() method is invoked only when its input arguments change. Pipes are pure by default.
In the transform() method, we can pass the array which needs to be filtered.
Code Explanation
In the SelectedItems array, we will filter the collection based on the selected property in the collection. If the selected property was setted to true means, it will filter into SelectedItems.
In the UnSelectedItems array, we will filter the collection based on the selected property in the collection. If the selected property was setted to false means, it will filter into UnSelectedItems.
Here I have used the spread operator (...) that will allow us to quickly copy all or part of an existing array or object into another array or object.
While returning, it will merge Selected and unselected items.
@Pipe({
name: 'orderByCheckboxSelection',
pure:false
})
export class CheckboxSelectionPipe implements PipeTransform {
transform(list: any): any {
if (!list) {
return [];
}
// Sort the items based on whether they are selected or not
let selectedItems = list.filter(item => item.selected);
let unselectedItems = list.filter(item => !item.selected);
return [...selectedItems, ...unselectedItems];
}
}
App.Module.ts for a particular module
Register the custom pipe in the module where you want to use it. For example, open the app.module.ts file and import and add CheckboxSelectionPipe to the declarations array.
@NgModule({
declarations: [....,....],
imports: [
CheckboxSelectionPipe
],
providers: [ ....]
App.Component.html
By providing the CheckBoxSelection pipe to the array needed to sort, you can sort the array based on that property's checkbox selection.
<div *ngFor="let date of collection | orderByCheckboxSelection ">
....
....
</div>