
October 3, 2024 08:08 by
Peter
I explained How to Show Data in a Dynamic Grid Using AngularJS in my earlier article. I'll explain in this article how to use AngularJS to apply CRUD operations in a dynamic grid. I previously shown to you how to display data in a grid format, but in this post, we'll take it a step further and enable editing of the grid; in other words, I'll be providing CRUD functions for the grid.
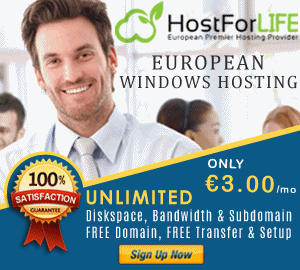
Step 1: Since I'm working on an earlier application, you may see where I left off in that post by reading it.
Now, I will update the JavaScript section and will provide the edit and delete functionality. For that, you need to write this code in the script section.
$scope.delete = function(id) {
for (i in $scope.employees) {
if ($scope.employees[i].id == id) {
$scope.employees.splice(i, 1);
$scope.newEmployee = {};
}
}
}
$scope.edit = function(id) {
for (i in $scope.employees) {
if ($scope.employees[i].id == id) {
$scope.newEmployee = angular.copy($scope.employees[i]);
}
}
}
First, I provided the delete functionality; for this, I have created a function named "delete". In this function the ID of the Employee is passed, if the ID correctly matches one of the existing IDs then the delete operation will be done and that entry will be deleted.
Similarly, I have created the "Edit" function. In this function, the ID of the Employee will also be passed, but this time the data related to that ID will not be deleted. Instead, it will be passed back to the corresponding textboxes so that the user can make changes, and when the user clicks the save button, the data will again be saved at the same Id position as it was stored previously.
Now, the updated Script looks like this.
<script>
var empid = 1;
function x($scope) {
$scope.employees = [
{ id: 0, 'name': 'Anubhav', 'address': 'Ghaziabad', 'dept': 'Developer' }
];
$scope.saveRecord = function() {
if ($scope.newEmployee.id == null) {
$scope.newEmployee.id = empid++;
$scope.employees.push($scope.newEmployee);
} else {
for (i in $scope.employees) {
if ($scope.employees[i].id == $scope.newEmployee.id) {
$scope.employees[i] = $scope.newEmployee;
}
}
}
$scope.newEmployee = {};
}
$scope.delete = function(id) {
for (i in $scope.employees) {
if ($scope.employees[i].id == id) {
$scope.employees.splice(i, 1);
$scope.newEmployee = {};
}
}
}
$scope.edit = function(id) {
for (i in $scope.employees) {
if ($scope.employees[i].id == id) {
$scope.newEmployee = angular.copy($scope.employees[i]);
}
}
}
}
</script>
Step 2. Now, I will work on the ViewModel of this application and will modify it as well.
You need to update your Table with this code.
<table border="1" bordercolor="blue">
<tr style="color:blue">
<th style="width:150px">Name</th>
<th style="width:150px">Address</th>
<th style="width:150px">Dept</th>
<th>Action</th>
</tr>
<tr style="color:pink" ng-repeat="employee in employees">
<td>{{ employee.name }}</td>
<td>{{ employee.address }}</td>
<td>{{ employee.dept }}</td>
<td>
<a href="#" ng-click="edit(employee.id)">edit</a> |
<a href="#" ng-click="delete(employee.id)">delete</a>
</td>
</tr>
</table>
Here, in the first Row, I added one more heading, "Action". In the second row, I added one more column in which two Anchors are used.
The first Anchor click is bound to the "Edit" function, and the second Anchor click is bound to the "Delete" function.
The ID of the Employee is passed depending on the Anchors, in other words wherever the Anchors are placed, the corresponding ID will be passed to the function.
Now our View Model is updated and its code is like this.
<div ng-app="" ng-controller="x">
<label>Name</label>
<input type="text" name="name" ng-model="newEmployee.name"/>
<label>Address</label>
<input type="text" name="address" ng-model="newEmployee.address"/>
<label>Dept.</label>
<input type="text" name="dept" ng-model="newEmployee.dept"/>
<br/>
<input type="hidden" ng-model="newEmployee.id"/>
<input type="button" value="Save" ng-click="saveRecord()" class="btn btn-primary"/>
<br/>
<br/>
<table border="1" bordercolor="blue">
<tr style="color:blue">
<th style="width:150px">Name</th>
<th style="width:150px">Address</th>
<th style="width:150px">Dept</th>
<th>Action</th>
</tr>
<tr style="color:pink" ng-repeat="employee in employees">
<td>{{ employee.name }}</td>
<td>{{ employee.address }}</td>
<td>{{ employee.dept }}</td>
<td>
<a href="#" ng-click="edit(employee.id)">edit</a> |
<a href="#" ng-click="delete(employee.id)">delete</a>
</td>
</tr>
</table>
</div>
Now, our application is created and is ready for execution.